go Cobra命令行工具入门
简介
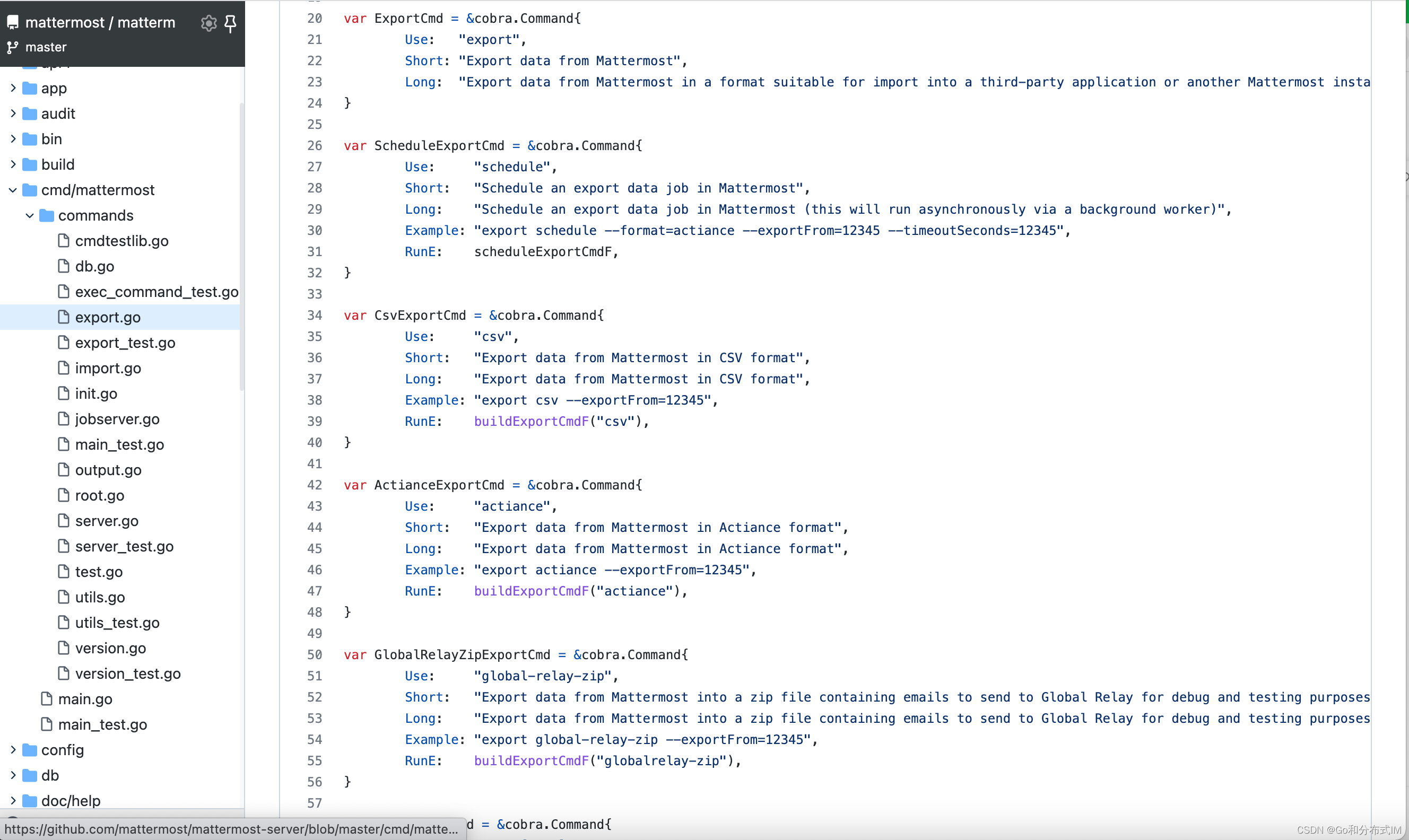
为什么需要cobra
使用前
package main import (
"flag"
"fmt"
) func main() {
flag.Parse() args := flag.Args()
if len(args) <= 0 {
fmt.Println("Usage: admin-cli [command]")
return
} switch args[0] {
case "help":
// ...
case "export":
//...
if len(args) == 3 { // 导出到文件
// todo
} else if len(args) == 2 { // 导出...
// todo
}
default:
//...
}
}
使用后
package main import (
"fmt"
"github.com/spf13/cobra"
"os"
) // rootCmd represents the base command when called without any subcommands
var rootCmd = &cobra.Command{
Use: "api",
Short: "A brief description of your application",
Long: `A longer description `,
} // 命令一
var mockMsgCmd = &cobra.Command{
Use: "mockMsg",
Short: "批量发送测试文本消息",
Long: ``,
Run: func(cmd *cobra.Command, args []string) {
fmt.Println("mockMsg called")
},
} // 命令二
var exportCmd = &cobra.Command{
Use: "export",
Short: "导出数据",
Long: ``,
Run: func(cmd *cobra.Command, args []string) {
fmt.Println("export called")
},
} func Execute() {
err := rootCmd.Execute()
if err != nil {
os.Exit(1)
}
} func init() {
rootCmd.Flags().BoolP("toggle", "t", false, "Help message for toggle") rootCmd.AddCommand(mockMsgCmd)
rootCmd.AddCommand(exportCmd) exportCmd.Flags().StringP("out", "k", "./backup", "导出路径")
} func main() {
Execute()
}
$ go run main.go
A longer description Usage:
api [command] Available Commands:
completion Generate the autocompletion script for the specified shell
export 导出数据
help Help about any command
mockMsg 批量发送测试文本消息 Flags:
-h, --help help for api
-t, --toggle Help message for toggle Use "api [command] --help" for more information about a command.
基本概念
- 命令(Commands ):代表行为。命令是程序的中心点,程序的每个功能都应该可以通过命令进行交互,一个命令可以有任意个子命令。
- 参数(Args):命令的参数
- 标志(Flags):修饰命令。它修饰命令该如何完成。
$ ./appName command args --Flag
- appName: hugo
- command: server
- flag: port
安装
Go pkg
$ go get -u github.com/spf13/cobra@latest
import "github.com/spf13/cobra"
命令行工具
# 命令行工具
$ go install github.com/spf13/cobra-cli@latest
$ cobra-cli --help
Cobra is a CLI library for Go that empowers applications.
This application is a tool to generate the needed files
to quickly create a Cobra application. Usage:
cobra-cli [command] Available Commands:
add Add a command to a Cobra Application
completion Generate the autocompletion script for the specified shell
help Help about any command
init Initialize a Cobra Application Flags:
-a, --author string author name for copyright attribution (default "YOUR NAME")
--config string config file (default is $HOME/.cobra.yaml)
-h, --help help for cobra-cli
-l, --license string name of license for the project
--viper use Viper for configuration Use "cobra-cli [command] --help" for more information about a command.
入门实践
新建cobra命令行程序
$ cobra-cli init
├── LICENSE
├── cmd
│ └── root.go
└── main.go
package main import "tools/api/cmd" func main() {
cmd.Execute()
}
root.go(有删减):
package cmd import (
"fmt" "github.com/spf13/cobra"
) // rootCmd represents the base command when called without any subcommands
var rootCmd = &cobra.Command{
Use: "api",
Short: "A brief description of your application",
Long: `A longer description `,
//Run: func(cmd *cobra.Command, args []string) {
// fmt.Println("api called")
//},
} // Execute adds all child commands to the root command and sets flags appropriately.
// This is called by main.main(). It only needs to happen once to the rootCmd.
func Execute() {
err := rootCmd.Execute()
if err != nil {
os.Exit(1)
}
} func init() {
// 全局flag
// rootCmd.PersistentFlags().StringVar(&cfgFile, "config", "", "config file (default is $HOME/.api.yaml)") // local flag,暂不知道用处
rootCmd.Flags().BoolP("toggle", "t", false, "Help message for toggle")
}
$ go build
$ ./api
A longer description Usage:
api [command] Available Commands:
completion Generate the autocompletion script for the specified shell
help Help about any command Flags:
-h, --help help for api
-t, --toggle Help message for toggle Use "api [command] --help" for more information about a command.
命令构成
- Available Commands:代表可以执行的命令。比如./api connect
- Flags:是参数。比如./api connect --ip=127.0.0.1:6379,--ip就是flag,127.0.0.1:6379就是flag的值。
新增命令
$ cobra-cli add [command]
$ cobra-cli add mock-msg
mockMsg created at /Users/xxx/repo/tools/api
package cmd import (
"fmt" "github.com/spf13/cobra"
) var mockMsgCmd = &cobra.Command{
Use: "mockMsg",
Short: "A brief description of your command",
Long: `mock msg command`,
Run: func(cmd *cobra.Command, args []string) {
fmt.Println("mockMsg called")
},
} func init() {
rootCmd.AddCommand(mockMsgCmd)
}
$ go build
$ ./api
// ...
Available Commands:
completion Generate the autocompletion script for the specified shell
help Help about any command
mockMsg A brief description of your command
// ...
$ ./api mockMsg mockMsg called
如何显示自己的命令用法
./api mockMsg help
批量生产mq消息 Usage:
benchmark mockmsg [flags] Flags:
-g, --goroutine int32 并发routine数量 (default 1)
-h, --help help for mockmsg
-p, --packet int32 每个routine一秒写入mq的数量 (default 20)
-g和-p是新增的2个flag:
func init() {
mockmsgCmd.Flags().Int32P("goroutine", "g", 1, "并发routine数量")
mockmsgCmd.Flags().Int32P("packet", "p", 20, "每个routine一秒写入mq的数量") rootCmd.AddCommand(mockmsgCmd)
}
// mockmsgCmd represents the mockmsg command
var mockmsgCmd = &cobra.Command{
Use: "mockmsg",
Short: "批量生产mq消息",
Run: func(cmd *cobra.Command, args []string) {
// 这里要写全名
g, _ := cmd.Flags().GetInt32("goroutine")
p, _ := cmd.Flags().GetInt32("packet")
fmt.Println("mockmsg called,flags:g=", g, ",p=", p, ",args:", args)
},
}
$ go run main.go mockmsg -p 322 -g 5 args1 args2
mockmsg called,flags:g= 5 ,p= 322 ,args: [args1 args2]
总结
- https://blog.csdn.net/qq_31639829/article/details/118889580
- https://github.com/mattermost/mattermost-server
go Cobra命令行工具入门的更多相关文章
- 探索Windows命令行系列(2):命令行工具入门
1.理论基础 1.1.命令行的前世今生 1.2.命令执行规则 1.3.使用命令历史 2.使用入门 2.1.启动和关闭命令行 2.2.执行简单的命令 2.3.命令行执行程序使用技巧 3.总结 1.理论基 ...
- [易学易懂系列|rustlang语言|零基础|快速入门|(25)|实战2:命令行工具minigrep(2)]
[易学易懂系列|rustlang语言|零基础|快速入门|(25)|实战2:命令行工具minigrep(2)] 项目实战 实战2:命令行工具minigrep 我们继续开发我们的minigrep. 我们现 ...
- [易学易懂系列|rustlang语言|零基础|快速入门|(24)|实战2:命令行工具minigrep(1)]
[易学易懂系列|rustlang语言|零基础|快速入门|(24)|实战2:命令行工具minigrep(1)] 项目实战 实战2:命令行工具minigrep 有了昨天的基础,我们今天来开始另一个稍微有点 ...
- 《Java从入门到失业》第二章:Java环境(三):Java命令行工具
2.3Java命令行工具 2.3.1编译运行 到了这里,是不是开始膨胀了,想写一段代码来秀一下?好吧,满足你!国际惯例,我们写一段HelloWorld.我们在某个目录下记事本,编写一段代码如下: 保存 ...
- .NET Core系列 : 1、.NET Core 环境搭建和命令行CLI入门
2016年6月27日.NET Core & ASP.NET Core 1.0在Redhat峰会上正式发布,社区里涌现了很多文章,我也计划写个系列文章,原因是.NET Core的入门门槛相当高, ...
- **代码审查:Phabricator命令行工具Arcanist的基本用法
Phabricator入门手册 http://www.oschina.net/question/191440_125562 Pharicator是FB的代码审查工具,现在我所在的团队也使用它来进行代码 ...
- 【No.2】监控Linux性能25个命令行工具
接着上一篇博文继续 [No.1]监控Linux性能25个命令行工具 10:mpstat -- 显示每个CPU的占用情况 该命令可以显示每个CPU的占用情况,如果有一个CPU占用率特别高,那么有可能是一 ...
- 【No.1】监控Linux性能25个命令行工具
如果你的Linux服务器突然负载暴增,告警短信快发爆你的手机,如何在最短时间内找出Linux性能问题所在?通过以下命令或者工具可以快速定位 top vmstat lsof tcpdump netsta ...
- NET Core 环境搭建和命令行CLI入门
NET Core 环境搭建和命令行CLI入门 2016年6月27日.NET Core & ASP.NET Core 1.0在Redhat峰会上正式发布,社区里涌现了很多文章,我也计划写个系列文 ...
随机推荐
- Linux shell中2>&1的含义解释
https://blog.csdn.net/zhaominpro/article/details/82630528
- Fastjsonfan反序列链学习前置知识
Fastjson前置知识 Fastjson 是一个 Java 库,可以将 Java 对象转换为 JSON 格式,当然它也可以将 JSON 字符串转换为 Java 对象. Fastjson 可以操作任何 ...
- css 实现图片专场
<template> <div> </div> </template> <style lang="scss"> html ...
- MySQL事务提交流程详解
MySQL事务的提交采用两阶段提交协议, 前些日子和同事聊的时候发现对提交的细节还是有些模糊,这里对照MySQL源码详细记录一下,版本是MySQL5.7.36. 一. 事务的提交流程. 1. 获取 M ...
- python黑帽子(第三章)
Windows/Linux下包的嗅探 根据os.name判断操作系统 下面是os的源码 posix是Linux nt是Windows 在windows中需要管理员权限.linux中需要root权限 因 ...
- oracle split 以及 简单json解析存储过程
BEGIN; 由于之前工作上需要在oracle中做split功能以及json格分解.然后经过一番google和优化整合,最后整理到一个存储过程包中,易于管理,代码如下: 1.包定义: CREATE O ...
- C#语法糖系列 —— 第一篇:聊聊 params 参数底层玩法
首先说说为什么要写这个系列,大概有两点原因. 这种文章阅读量确实高... 对 IL 和 汇编代码 的学习巩固 所以就决定写一下这个系列,如果大家能从中有所收获,那就更好啦! 一:params 应用层玩 ...
- vue动态路由实现原理 addRoute
vue新版router.addRoute基础用法 新版Vue Router中用router.addRoute来替代原有的router.addRoutes来动态添加路由.子路由 在添加子路由的时候 比如 ...
- 记一次 PHP 省市县三级联动 数据库取值
/** * Notes:省市县三级联动 * Created by depressiom * Date: 2022年4月14日 */ public function getCityData(){ //获 ...
- react实战系列 —— 我的仪表盘(bizcharts、antd、moment)
其他章节请看: react实战 系列 My Dashboard 上一篇我们在 spug 项目中模仿"任务计划"模块实现一个类似的一级导航页面("My任务计划") ...