POJ2286 The Rotation Game
Description
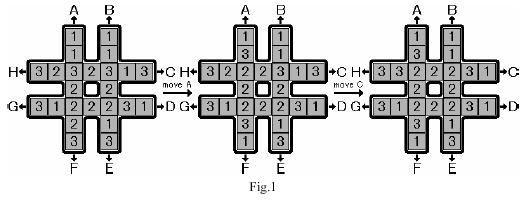
Initially, the blocks are placed on the board randomly. Your task is to move the blocks so that the eight blocks placed in the center square have the same symbol marked. There is only one type of valid move, which is to rotate one of the four lines, each consisting of seven blocks. That is, six blocks in the line are moved towards the head by one block and the head block is moved to the end of the line. The eight possible moves are marked with capital letters A to H. Figure 1 illustrates two consecutive moves, move A and move C from some initial configuration.
Input
Output
Sample Input
1 1 1 1 3 2 3 2 3 1 3 2 2 3 1 2 2 2 3 1 2 1 3 3
1 1 1 1 1 1 1 1 2 2 2 2 2 2 2 2 3 3 3 3 3 3 3 3
0
Sample Output
AC
2
DDHH
2
Source
正解:迭代加深搜索(+大剪枝)
解题报告:
北大信息学夏令营一试第一题,当时考场上看没什么人做于是果断没做,考完再看才发现是个搜索题。
今天考试居然考到了这道题,好吧,就来填这个坑吧。
首先迭代加深,确定搜索深度上界(保证dfs不会gi),然后我们考虑各种奇奇怪怪的剪枝。
显然,我们会在最终得到中间的一圈全是1或2或3,不妨取一个目前已经数量最多的数字来做一个估价若已经超过上界,就直接返回。
还有一个剪枝,如果上一次往上移动,那么这一次就不要往下移动。
代码如下:
//It is made by jump~
#include <iostream>
#include <cstdlib>
#include <cstring>
#include <cstdio>
#include <cmath>
#include <algorithm>
#include <ctime>
#include <vector>
#include <queue>
#include <map>
#ifdef WIN32
#define OT "%I64d"
#else
#define OT "%lld"
#endif
using namespace std;
typedef long long LL;
/*
图形一览:
0 1
2 3
4 5 6 7 8 9 10
11 12
13 14 15 16 17 18 19
20 21
22 23
*/
int num[][]={{,,,,,,},{,,,,,,},{,,,,,,},{,,,,,,}};
int ying[]={,,,,,,,};
int center[]={,,,,,,,};
int a[];
int ans,sh;
char out[]; inline int getint()
{
int w=,q=;
char c=getchar();
while((c<'' || c>'') && c!='-') c=getchar();
if (c=='-') q=, c=getchar();
while (c>='' && c<='') w=w*+c-'', c=getchar();
return q ? -w : w;
} inline bool check(){
int xun=a[center[]];
for(int i=;i<=;i++) if(a[center[i]]!=xun) return false;
return true;
} inline void mov(int x){
int cun=a[num[x][]];
for(int i=;i<=;i++) {
a[num[x][i]]=a[num[x][i+]];
}
a[num[x][]]=cun;
} inline int count(int x){
int total=;
for(int i=;i<=;i++) if(a[center[i]]!=x) total++;
return total;
} inline int gu(){
return min( min(count(),count()) , count());
} inline void init(){
for(int i=;i<=;i++) for(int j=;j<=;j++) num[i][j]=num[ying[i]][-j];
ying[]=;
} inline bool dfs(int tim,int limit,int last){
if(check()){
//out[tim]=a[center[0]];
sh=a[center[]];
return true;
}
else {
if(gu()+tim>limit) return false;
for(int i=;i<;i++) {
if(ying[last]==i) continue;
out[tim]='A'+i;
mov(i);
if(dfs(tim+,limit,i)) return true;
mov(ying[i]);
}
}
return false;
} inline void solve(){
init();
while(){
a[]=getint();
if(!a[]) break;
for(int i=;i<=;i++) a[i]=getint(); if(check()) printf("No moves needed\n%d\n",a[center[]]);
else{
for(ans=;;ans++) {
if(dfs(,ans,)) break;
}
printf("%s",out);
printf("\n%d\n",sh);
}
memset(out,'\0',sizeof(out));
}
} int main()
{
solve();
return ;
}
POJ2286 The Rotation Game的更多相关文章
- [poj2286]The Rotation Game (IDA*)
//第一次在新博客里发文章好紧张怎么办 //MD巨神早已在一个小时前做完了 The Rotation Game Time Limit: 15000MS Memory Limit: 150000K To ...
- POJ2286 The Rotation Game(IDA*)
The Rotation Game Time Limit: 15000MS Memory Limit: 150000K Total Submissions: 5691 Accepted: 19 ...
- POJ2286 The Rotation Game[IDA*迭代加深搜索]
The Rotation Game Time Limit: 15000MS Memory Limit: 150000K Total Submissions: 6325 Accepted: 21 ...
- A*专题训练
POJ2449 Remmarguts' Date UDF's capital consists of N stations. The hall is numbered S, while the sta ...
- POJ2286:The Rotation Game——题解
http://poj.org/problem?id=2286 题目大意:如图所示有一种玩具,每次可以拉动A-H的开关使得整个行(或列)向字母方向移动一位(如果移动到头的话则到行(列)尾部) 求使得中间 ...
- UVALive 7139 Rotation(矩阵前缀和)(2014 Asia Shanghai Regional Contest)
题目链接:https://icpcarchive.ecs.baylor.edu/index.php?option=com_onlinejudge&Itemid=8&category=6 ...
- The Rotation Game(IDA*算法)
The Rotation Game Time Limit : 30000/15000ms (Java/Other) Memory Limit : 300000/150000K (Java/Othe ...
- unity3d 的Quaternion.identity和transform.rotation区别是什么
Quaternion.identity就是指Quaternion(0,0,0,0),就是每旋转前的初始角度,是一个确切的值,而transform.rotation是指本物体的角度,值是不确定的,比如可 ...
- ios layer 动画-(transform.rotation篇)
x轴旋转: CABasicAnimation *theAnimation; theAnimation=[CABasicAnimation animationWithKeyPath:@"tra ...
随机推荐
- Windows远程桌面连接Ubuntu 14.04
由于xrdp.gnome和unity之间的兼容性问题,在Ubuntu 14.04版本中仍然无法使用xrdp登陆gnome或unity的远程桌面,现象是登录后只有黑白点为背景,无图标也无法操作.与13. ...
- Spring 中注入 properties 中的值
<bean id="ckcPlaceholderProperties" class="org.springframework.beans.factory.confi ...
- 关于 app测试工具
1. 腾讯的GT 2. testin 云测 3. monkey的自动化测试 4. 纯手工的功能测试.
- result默认返回action中的所有数据,要想返回指定的数据怎么做呢
result默认返回action中的所有数据,要想返回指定的数据怎么做呢?
- 1445 送Q币
1445 送Q币 时间限制: 1 s 空间限制: 1000 KB 题目等级 : 钻石 Diamond 题解 查看运行结果 题目描述 Description 一次在玩网络游戏的过程中,在 ...
- Navi.Soft20.WinCE使用手册
1.概述 1.1应用场景 随着物联网的普及,越来越多的制造商对货品从原料配备,加工生产,销售出库等环节的要求和把控越来越高.在此情况之下,传统的ERP软件已经无法满足现有的流程. 移动设备的应用,在很 ...
- 安装mysql-connector-python
安装mysql-connector-python 1.下载. wget http://dev.mysql.com/get/Downloads/Connector-Python/mysql-connec ...
- MVC3学习:利用mvc3+ajax实现登录
用到的工具或技术:vs2010,EF code first,JQuery ajax,mvc3. 第一步:准备数据库. 利用EF code first,先写实体类,然后根据实体类自动创建数据库:或者先创 ...
- Ubuntu 16.04 LTS安装好需要设置的15件事(喜欢新版本)
看到这篇文章说明你已经从老版本升级到 Ubuntu 16.04 或进行了全新安装,在安装好 Ubuntu 16.04 LTS 之后建议大家先做如下 15 件事.无论你是刚加入 Ubuntu 行列的新用 ...
- 回顾一年的IT学习历程与大学生活
今天是2015年8月27日,距离成为大三狗还有一个多星期,在这个不算繁忙的暑假的下午来总结一下这一年来,在IT方面的学习. 一.入门(2014.3) 我大一的专业是信息工程,信息工程听上去就是信息(I ...