AtCoder Beginner Contest 086 (ABCD)
A - Product
题目链接:https://abc086.contest.atcoder.jp/tasks/abc086_a
Time limit : 2sec / Memory limit : 256MB
Score : 100 points
Problem Statement
AtCoDeer the deer found two positive integers, a and b. Determine whether the product of a and b is even or odd.
Constraints
- 1 ≤ a,b ≤ 10000
- a and b are integers.
Input
Input is given from Standard Input in the following format:
a b
Output
If the product is odd, print Odd
; if it is even, print Even
.
Sample Input 1
3 4
Sample Output 1
Even
As 3×4=12 is even, print Even
.
Sample Input 2
1 21
Sample Output 2
Odd
As 1×21=21 is odd, print Odd
.
#include<bits/stdc++.h>
using namespace std; int main()
{
int a,b;
while(cin>>a>>b){
if((a*b)%==) cout<<"Even"<<endl;
else cout<<"Odd"<<endl;
}
return ;
}
B - 1 21
题目链接:https://abc086.contest.atcoder.jp/tasks/abc086_b
Time limit : 2sec / Memory limit : 256MB
Score : 200 points
Problem Statement
AtCoDeer the deer has found two positive integers, a and b. Determine whether the concatenation of a and b in this order is a square number.
Constraints
- 1 ≤ a,b ≤ 100
- a and b are integers.
Input
Input is given from Standard Input in the following format:
a b
Output
If the concatenation of a and b in this order is a square number, print Yes
; otherwise, print No
.
Sample Input 1
1 21
Sample Output 1
Yes
As 121 = 11 × 11, it is a square number.
Sample Input 2
100 100
Sample Output 2
No
100100 is not a square number.
Sample Input 3
12 10
Sample Output 3
No
题解:直接判断是否能开方
#include<bits/stdc++.h>
using namespace std;
bool prime(int x)
{
int y=sqrt(x);
return y*y==x;
}
int main()
{
int a,b;
while(cin>>a>>b){
if(a<b) swap(a,b);
int flag=;
if(a<&&b<){
if(prime(a+*b)||prime(a*+b)) flag=;
}
else if(a>=&&a<&&b<){
if(prime(a+*b)||prime(a*+b)) flag=;
}
else if(a>=&&a<&&b>=&&b<){
if(prime(a+*b)||prime(a+*b)) flag=;
}
else if(a==&&b>=&&b<){
if(prime(a+*b)||prime(a*+b)) flag=;
}
if(flag) cout<<"Yes"<<endl;
else cout<<"No"<<endl;
}
return ;
}
C - Traveling
题目链接:https://abc086.contest.atcoder.jp/tasks/arc089_a
C - Traveling
Time limit : 2sec / Memory limit : 256MB
Score : 300 points
Problem Statement
AtCoDeer the deer is going on a trip in a two-dimensional plane. In his plan, he will depart from point (0,0) at time 0, then for each i between 1 and N (inclusive), he will visit point (xi,yi) at time ti.
If AtCoDeer is at point (x,y) at time t, he can be at one of the following points at time t+1: (x+1,y), (x−1,y), (x,y+1) and (x,y−1). Note that he cannot stay at his place. Determine whether he can carry out his plan.
Constraints
- 1 ≤ N ≤ 105
- 0 ≤ xi ≤ 105
- 0 ≤ yi ≤ 105
- 1 ≤ ti ≤ 105
- ti < ti+1 (1 ≤ i ≤ N−1)
- All input values are integers.
Input
Input is given from Standard Input in the following format:
N
t1 x1 y1
t2 x2 y2
:
tN xN yN
Output
If AtCoDeer can carry out his plan, print Yes
; if he cannot, print No
.
Sample Input 1
2
3 1 2
6 1 1
Sample Output 1
Yes
For example, he can travel as follows: (0,0), (0,1), (1,1), (1,2), (1,1), (1,0), then (1,1).
Sample Input 2
1
2 100 100
Sample Output 2
No
It is impossible to be at (100,100) two seconds after being at (0,0).
Sample Input 3
2
5 1 1
100 1 1
Sample Output 3
No 题解:判断纵坐标和横坐标之后是否大于t 如果大于再减去t判断是否能够被我整除
#include<bits/stdc++.h>
using namespace std;
bool test(int t,int x,int y)
{
int z=x+y;
if(t<z) return ;
else{
if((t-z)%==) return ;
else return ;
}
}
int main()
{
int n;
while(cin>>n){
int t,x,y;
int flag=;
while(n--){
cin>>t>>x>>y;
if(!test(t,x,y)) flag=;
}
if(flag) cout<<"Yes"<<endl;
else cout<<"No"<<endl;
}
return ;
}
D - Checker (前缀和)
题目链接:https://abc086.contest.atcoder.jp/tasks/arc089_b
Time limit : 2sec / Memory limit : 256MB
Score : 500 points
Problem Statement
AtCoDeer is thinking of painting an infinite two-dimensional grid in a checked pattern of side K. Here, a checked pattern of side K is a pattern where each square is painted black or white so that each connected component of each color is a K × K square. Below is an example of a checked pattern of side 3:
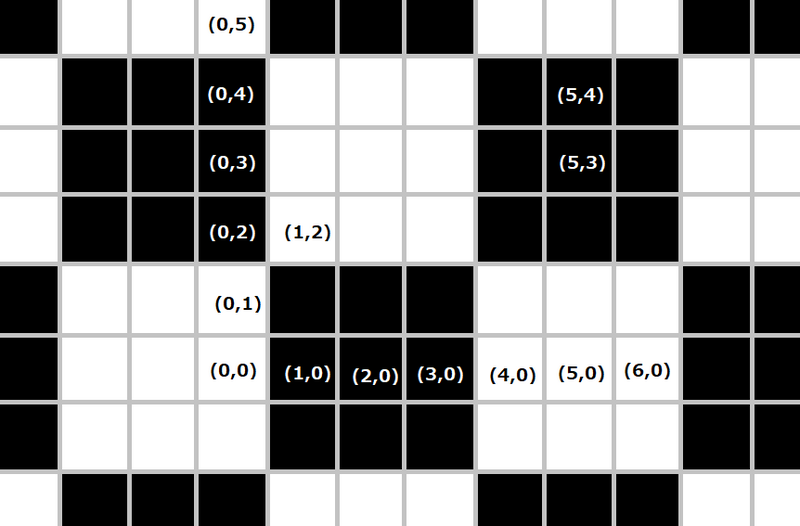
AtCoDeer has N desires. The i-th desire is represented by xi, yi and ci. If ci is B
, it means that he wants to paint the square (xi,yi) black; if ci is W
, he wants to paint the square (xi,yi) white. At most how many desires can he satisfy at the same time?
Constraints
- 1 ≤ N ≤ 105
- 1 ≤ K ≤ 1000
- 0 ≤ xi ≤ 109
- 0 ≤ yi ≤ 109
- If i ≠ j, then (xi,yi) ≠ (xj,yj).
- ci is
B
orW
. - N, K, xi and yi are integers.
Input
Input is given from Standard Input in the following format:
N K
x1 y1 c1
x2 y2 c2
:
xN yN cN
Output
Print the maximum number of desires that can be satisfied at the same time.
Sample Input 1
4 3
0 1 W
1 2 W
5 3 B
5 4 B
Sample Output 1
4
He can satisfy all his desires by painting as shown in the example above.
Sample Input 2
2 1000
0 0 B
0 1 W
Sample Output 2
2
Sample Input 3
6 2
1 2 B
2 1 W
2 2 B
1 0 B
0 6 W
4 5 W
Sample Output 3
4
题解:思路是对的 但是暴力过不了 看看大佬的解法 转自:https://www.cnblogs.com/8023spz/p/8409399.html
这道题数据挺大的,首先是移动到2k*2k的框框里,不影响坐标(也就是横纵坐标都移动2k的整数倍,如果只移动k的整数倍不能保证颜色不变)。
最初先默认数黑色或者白色都可以,这里先默认数黑色,白色的通过变色移动(横或纵坐标+k)映射到黑色的位置,然后整个图里只剩下黑色的。
最后求出结果,只需要取sum 和 n - sum中大的那个就行了,因为默认是黑色,黑白可以互换。
然后是移动k宫格在不同位置,一共有k*k种情况,直接在2k*2k的框框里操作,需要计算二维前缀和,之前是一个一个点去判断超时了,想想也不能一味的暴力啊,肯定得用点妙招,然后移动x轴,计算一维前缀和时间勉强没超。
k宫格是从左下角开始移动就是从(0,0)开始,需要记录的是k宫格里黑色的个数,以及斜对角的矩形里黑色的个数,因为斜对角的颜色相同,横竖相邻的区域颜色相异。
计算二维前缀和需要用离散学的容斥定理,最后求单独区域需要前缀和相减,也要用到容斥定理。
#include <iostream>
#include <algorithm>
#include <cstdio>
#include <cstdlib>
#include <cstring>
#include <cmath>
#include <iomanip> using namespace std; int v[][];
int n,k;
int num(int x1,int y1,int x2,int y2){
return v[x2][y2] - v[x2][y1] - v[x1][y2] + v[x1][y1];///前缀和 减去左边 下边和左下边的k宫格前缀和 容斥定理:左边和下边都包含了左下边 需要加上一个左下边
}
int main()
{
int x,y,ans = ,sum;
char ch;
scanf("%d%d",&n,&k);
for(int i = ;i <= n;i ++){
scanf("%d%d %c",&x,&y,&ch);
x %= * k;///把所有点都移动到 2k * 2k 的区域
y %= * k;
x += k * (ch == 'W');///x可以变成y W 也可以变成 B 这里白色都变成黑色的 如果转换后的黑色是满足的那么原来的白色也一定是满足的
v[x % ( * k) + ][y % ( * k) + ] ++;///求前缀和要求从(1,1)开始
}
for(int i = ;i <= * k;i ++)///求前缀和
for(int j = ;j <= * k;j ++)
v[i][j] += v[i - ][j] + v[i][j - ] - v[i - ][j - ];///加上左边下边和左下角的和 容斥定理:左边和下边的都包含了左下边的 要减去一个左下边的for(int i = 1;i <= k;i++)///k * k个格子依次做为起点构成新的k宫格 也就是移动k宫格 看看有几个黑色点包含在内 (默认黑色 可互换)
for(int j = 1;j <= k;j++)
{
///斜对角的k宫格是相同颜色 2k * 2k区域最多有五个这样的区域
sum = num(,,i,j) + num(i,j,k + i,k + j) + num(k + i,k + j, * k, * k) + num(k + i,, * k,j) + num(,k + j,i, * k);
ans = max(ans,max(sum,n - sum));///黑白色可以互换
}
printf("%d\n",ans);
}
AtCoder Beginner Contest 086 (ABCD)的更多相关文章
- AtCoder Beginner Contest 085(ABCD)
A - Already 2018 题目链接:https://abc085.contest.atcoder.jp/tasks/abc085_a Time limit : 2sec / Memory li ...
- AtCoder Beginner Contest 254(D-E)
Tasks - AtCoder Beginner Contest 254 D - Together Square 题意: 给定一个N,找出所有不超过N的 ( i , j ),使得( i * j )是一 ...
- AtCoder Beginner Contest 084(AB)
A - New Year 题目链接:https://abc084.contest.atcoder.jp/tasks/abc084_a Time limit : 2sec / Memory limit ...
- AtCoder Beginner Contest 083 (AB)
A - Libra 题目链接:https://abc083.contest.atcoder.jp/tasks/abc083_a Time limit : 2sec / Memory limit : 2 ...
- AtCoder Beginner Contest 264(D-E)
D - "redocta".swap(i,i+1) 题意: 给一个字符串,每次交换相邻两个字符,问最少多少次变成"atcoder" 题解: 从左到右依次模拟 # ...
- Atcoder Beginner Contest 155E(DP)
#definde HAVE_STRUCT_TIMESPEC #include<bits/stdc++.h> using namespace std; ]; int main(){ ios: ...
- Atcoder Beginner Contest 121D(异或公式)
#include<bits/stdc++.h>using namespace std;int main(){ long long a,b; cin>>a>&g ...
- Atcoder Beginner Contest 156E(隔板法,组合数学)
#define HAVE_STRUCT_TIMESPEC #include<bits/stdc++.h> using namespace std; ; ; long long fac[N] ...
- Atcoder Beginner Contest 155D(二分,尺取法,细节模拟)
二分,尺取法,细节模拟,尤其是要注意a[i]被计算到和a[i]成对的a[j]里时 #define HAVE_STRUCT_TIMESPEC #include<bits/stdc++.h> ...
随机推荐
- centos安装Django之一:安装openssl
这几天在部署Django,需要安装的东西有点多,python3.pip3.openssl(pip依赖ssl环境),所以第一步是安装openssl,如何安装呢?主要有三步,随ytkah一起来看看吧 1. ...
- 自动化工具之二:win32gui
自动化工具win32gui 一.下载安装win32gui 二.Win32gui的使用 1.查找窗体句柄 我们知道的所有空间其实就是窗体,所有的窗口都有一个独立的句柄,要操作任意一个窗体,你都需要找到这 ...
- Kibana5.x界面简要介绍(含x-pack插件)
简介:Kibana是一个为 ElasticSearch 提供的数据分析的 Web 接口(5601).可使用它对日志进行高效的搜索.可视化.分析等各种操作.Kibana目前最新的版本5.3.X-Pack ...
- EntityFrameworkCore概览
.NET Core 中 EntityFrameworkCore的支持库主要有: Script-migration 级联删除 protected override void OnConfiguring( ...
- mac控制台快捷键
ctrl+a //移到行首ctrl+e //移到行尾 ctrl+y // 插入最近删除的单词或语句ctrl+k //删除光标处到行尾部分ctrl+u //删除光标处到行首部分ctrl+w //删除光标 ...
- Bug 5323844-IMPDP无法导入远程数据库同义词的同义词
参见MOS文档: Bug 5323844 - SYNONYM for a SYNONYM in remote database not imported using IMPDP (文档 ID 5323 ...
- react结合redux开发
先加上我码云的地址:https://gitee.com/ldlx/react_and_rudex
- React-Native组件之Text内文字垂直居中方案
style: { height: 100, textAlign: 'center', textAlignVertical: 'center', } 以上方法在Android上显示水平垂直居中, 但在I ...
- DataGridView控件用法一:数据绑定
使用DataGridView控件,可以显示和编辑来自多种不同类型的数据源的表格数据. 将数据绑定到DataGridView控件非常简单和直观,在大多数情况下,只需设置DataSource属性即可.在绑 ...
- Semaphore wait has lasted > 600 seconds
解决方案:set global innodb_adaptive_hash_index=0;