Leetcode solution 291: Word Pattern II
Problem Statement
pattern
and a string str
, find if str
follows the same pattern.pattern
and a non-empty substring in str
.Input: pattern ="abab"
, str ="redblueredblue"
Output: true
Input: pattern = pattern ="aaaa"
, str ="asdasdasdasd"
Output: true
Input: pattern ="aabb"
, str ="xyzabcxzyabc"
Output: false
You may assume both
pattern
and str
contains only lowercase letters.Problem link
Video Tutorial
You can find the detailed video tutorial here
- Youtube
- B站
Thought Process
It is quite similar to Word Pattern and Isomorphic String problem, where we would keep a mapping from char to a string while also ensure there would be a one to one mapping, i.e., bijection mapping. The tricky part is it seems there are way many combinations of the mapping, how can we efficiently solve them?
Maybe we could list all the combinations? Maybe we could use DP since it is string related and only ask for true/false result?
How to list all combinations? Think about this way, let's say you have pattern = "aba" and str = "redbluered", since one char in pattern can map to any string length >= 1 in str, it is equivalent to divide up str into 3 parts (length of pattern) and check all cases. For instance, the cut of the words is like below:
- r | e | d b l u e r e d
- r | e d | b l u e r e d
- r | e d b | l u e r e d
- r | e d b l | u e r e d
- r | e d b l u | e r e d
- r | e d b l u e | r e d
- r | e d b l u e r | e d
- r | e d b l u e r e | d
- r e | d | b l u e r e d
- r e | d b | l u e r e d
- r e | d b l | u e r e d
- r e | d b l u | e r e d
- r e | d b l u e | r e d
- r e | d b l u e r | e d
- r e | d b l u e r e | d
- r e d | b | l u e r e d
- .....
In general, if the length of pattern is M, the str length is N, the time complexity of this brute force method is O(N^M), more accurately, it should be
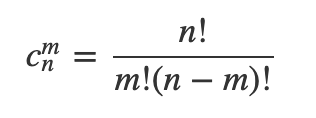
DP solution does not work since we cannot easily get a deduction formula :(
Solutions
Brute force list all the combos
For each character in pattern, try to map any possible remaining strings in str from length 1 to the end. During this process, need to make sure the string mapping is bijection (no two chars in pattern map to the same string in str) and if a mapping has been seen before, continue use that mapping
A DFS recursion would be the implementation. A few caveats in implementation
- Remember to reset the map and set after recursion returned false
- When there is a bijection mapping, should continue instead of directly break
public boolean wordPatternMatch(String pattern, String str) {
if (pattern == null || str == null) {
return false;
} Map<Character, String> lookup = new HashMap<>();
Set<String> dup = new HashSet<>(); return this.isMatch(pattern, str, lookup, dup);
} // DFS recursion to list out all the possibilities
public boolean isMatch(String pattern, String str, Map<Character, String> lookup, Set<String> dup) {
if (pattern.length() == 0) {
return str.length() == 0;
} char c = pattern.charAt(0); if (lookup.containsKey(c)) {
String mappedString = lookup.get(c);
if (mappedString.length() > str.length()) {
return false;
} // could use str.startsWith(mappedString)
if (!mappedString.equals(str.substring(0, mappedString.length()))) {
return false;
} return this.isMatch(pattern.substring(1), str.substring(mappedString.length()), lookup, dup); } else {
for (int i = 1; i <= str.length(); i++) {
String mappingString = str.substring(0, i);
if (dup.contains(mappingString)) {
// not a bijection mapping, not not return false, but continue
continue;
} lookup.put(c, mappingString);
dup.add(mappingString);
if (this.isMatch(pattern.substring(1), str.substring(i), lookup, dup)) {
return true;
}
// reset value for next recursion iteration for backtracking
lookup.remove(c);
dup.remove(mappingString);
}
} return false;
}
Time Complexity: O(N^M), or C(N^M) to be exact. Pattern length is M, str length is N
Space Complexity: O(M), Pattern length is M, str length is N. We use a map and a set to store the lookup, but at one time, the map should not exceed the pattern size, so is the set
References
Leetcode solution 291: Word Pattern II的更多相关文章
- [LeetCode] 291. Word Pattern II 词语模式 II
Given a pattern and a string str, find if str follows the same pattern. Here follow means a full mat ...
- 291. Word Pattern II
题目: Given a pattern and a string str, find if str follows the same pattern. Here follow means a full ...
- Baozi Leetcode Solution 290: Word Pattern
Problem Statement Given a pattern and a string str, find if str follows the same pattern. Here follo ...
- leetcode 290. Word Pattern 、lintcode 829. Word Pattern II
290. Word Pattern istringstream 是将字符串变成字符串迭代器一样,将字符串流在依次拿出,比较好的是,它不会将空格作为流,这样就实现了字符串的空格切割. C++引入了ost ...
- Word Pattern | & II
Word Pattern | Given a pattern and a string str, find if str follows the same pattern. Examples: pat ...
- Word Pattern II 解答
Question Given a pattern and a string str, find if str follows the same pattern. Here follow means a ...
- leetcode面试准备: Word Pattern
leetcode面试准备: Word Pattern 1 题目 Given a pattern and a string str, find if str follows the same patte ...
- [LeetCode] Word Pattern II 词语模式之二
Given a pattern and a string str, find if str follows the same pattern. Here follow means a full mat ...
- 【LeetCode OJ】Word Break II
Problem link: http://oj.leetcode.com/problems/word-break-ii/ This problem is some extension of the w ...
随机推荐
- Android零基础入门第73节:Activity初入门,创建和配置如此简单
Activity是Android应用的重要组成单元之一,也是Android应用最常见的组件之一.前面看到的示例通常都只包含一个Activity或一个AppCompatActivity,但在实际应用中这 ...
- CKEditor 4.5 beta 发布,可视化 HTML 编辑器
分享 <关于我> 分享 [中文纪录片]互联网时代 http://pan.baidu.com/s/1qWkJfcS 分享 <HTML开发MacOSAp ...
- Delphi开发 Android 程序启动画面简单完美解决方案
原文在这里 还是这个方法好用,简单!加上牧马人做的自动生成工具,更是简单. 以下为原文,向波哥敬礼! 前面和音儿一起研究 Android 下启动画面的问题,虽然问题得到了解决,但是,总是感觉太麻烦,主 ...
- 抓取报表ALV GRID上的数据
在项目开发过程中需要从标准报表MB5B中获取数据,以下是本人实例中的相关部分,程序同样适用于获取其他标准报表的数据. CL_SALV_BS_RUNTIME_INFO=>SET( DISPL ...
- js数字转成金额格式
本文有以下三个段落 1.方法展示 2.方法说明 3.方法实例 1.方法展示 //将数字转换成金额显示 function toMoney(num){ num = num.toFixed(2); num ...
- ABP开发框架前后端开发系列---(7)系统审计日志和登录日志的管理
我们了解ABP框架内部自动记录审计日志和登录日志的,但是这些信息只是在相关的内部接口里面进行记录,并没有一个管理界面供我们了解,但是其系统数据库记录了这些数据信息,我们可以为它们设计一个查看和导出这些 ...
- 算法与数据结构基础 - 堆栈(Stack)
堆栈基础 堆栈(stack)具有“后进先出”的特性,利用这个特性我们可以用堆栈来解决这样一类问题:后续的输入会影响到前面的阶段性结果.线性地遍历输入并用stack处理,这类问题较简单,求解时间复杂度一 ...
- python模块之:paramiko
1. 介绍: paramiko是一个用于做远程控制的模块,使用该模块可以对远程服务器进行命令或文件操作,值得一说的是,fabric和ansible内部的远程管理就是使用的paramiko来实现.安装: ...
- Confluence迁移
本次操作系统版本Centos7.3,Confluence版本5.9.9. 一.数据迁移 1.在旧Confluence上打包 “confluence和confluence-data”整个目录,默认安装的 ...
- C++类的完美单元测试方案——基于C++11扩展的friend语法
版权相关声明:本文所述方案来自于<深入理解C++11—C++11新特性解析与应用>(Michael Wong著,机械工业出版社,2016.4重印)一书的学习. 项目管理中,C语言工程做单元 ...