ExpandableListView之BaseExpandableListAdapter
之前使用的SimpleExpandableListAdapter有较大局限性,样式单一,修改难度大,这里不建议使用,而是利用BaseExpandableListAdapter,其实SimpleExpandableListAdapter也是继承自BaseExpandableListAdapter,这里用到的布局和上一个一样,先定义一个内部类MyExpandableListViewAdapter继承自BaseExpandableListAdapter,需要实现几个方法:
1.getGroupCount() 返回父元素的个数
2.getChildrenCount(int groupPosition) 返回当前父元素下的子元素个数
3.getGroup(int groupPosition) 返回当前父元素的数据
4.getChild(int groupPosition, int childPosition) 返回当前父元素下子元素的数据
5.getGroupId(int groupPosition) 返回当前父元素的id
6.getChildId(int groupPosition, int childPosition) 返回当前父元素下的子元素的id
7.hasStableIds() 是否有稳定的id,这里默认false不修改
8.getGroupView(int groupPosition, boolean isExpanded, View convertView, ViewGroup parent) 返回当前父元素的view
9.getChildView(int groupPosition, int childPosition, boolean isLastChild, View convertView, ViewGroup parent) 返回当前子元素的view
10.isChildSelectable(int groupPosition, int childPosition) 是否可以选择,默认false,这里用不到,不修改
SecondActivity:
package com.fitsoft;
import android.graphics.Color;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.util.TypedValue;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.BaseExpandableListAdapter;
import android.widget.ExpandableListView;
import android.widget.TextView;
public class SecondActivity extends AppCompatActivity {
ExpandableListView expandableListView;
MyExpandableListViewAdapter myExpandableListViewAdapter;
String[] groupStringArr = {"腾讯", "百度", "阿里巴巴"};
String[][] childStringArr = {
{"QQ","微信","QQ浏览器"},
{"百度搜索","百度地图","百度外卖"},
{"淘宝","支付宝","天猫商城"}
};
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
expandableListView = findViewById(R.id.expandable_ListView);
myExpandableListViewAdapter = new MyExpandableListViewAdapter();
expandableListView.setAdapter(myExpandableListViewAdapter);
}
class MyExpandableListViewAdapter extends BaseExpandableListAdapter {
@Override
public int getGroupCount() {
return groupStringArr.length;
}
@Override
public int getChildrenCount(int groupPosition) {
return childStringArr[groupPosition].length;
}
@Override
public Object getGroup(int groupPosition) {
return groupStringArr[groupPosition];
}
@Override
public Object getChild(int groupPosition, int childPosition) {
return childStringArr[groupPosition][childPosition];
}
@Override
public long getGroupId(int groupPosition) {
return groupPosition * 100;
}
@Override
public long getChildId(int groupPosition, int childPosition) {
return groupPosition * 100 + childPosition;
}
@Override
public boolean hasStableIds() {
return false;
}
@Override
public View getGroupView(int groupPosition, boolean isExpanded, View convertView, ViewGroup parent) {
View view = convertView;
if(view == null){
view = LayoutInflater.from(getApplicationContext()).inflate(R.layout.item_group, parent, false);
}
Object data = getGroup(groupPosition);
TextView textView = view.findViewById(R.id.tv_group);
textView.setText(data.toString());
if(groupPosition % 2 == 1){
textView.setTextColor(Color.RED);
}else{
textView.setTextColor(Color.BLUE);
}
return view;
}
@Override
public View getChildView(int groupPosition, int childPosition, boolean isLastChild, View convertView, ViewGroup parent) {
View view = convertView;
if(view == null){
view = LayoutInflater.from(getApplicationContext()).inflate(R.layout.item_child, parent, false);
}
TextView textView = view.findViewById(R.id.tv_child);
Object data = getChild(groupPosition, childPosition);
textView.setText(data.toString());
if(childPosition > 1){
textView.setTextSize(TypedValue.COMPLEX_UNIT_SP, 20);
}else{
textView.setTextSize(TypedValue.COMPLEX_UNIT_SP, 15);
}
return view;
}
@Override
public boolean isChildSelectable(int groupPosition, int childPosition) {
return false;
}
}
}
这里的数据源用的是上一个数据源,主要实现的方法是getGroupView和getChildView,另外要注意的是,设置id的时候,为避免父元素和子元素id重复,设置父元素位置*100为其真正id,这样只要子元素不超过100个,就不会重复。
View view = convertView;
if(view == null){
view = LayoutInflater.from(getApplicationContext()).inflate(R.layout.item_group, parent, false);
}
利用这几句代码判断当前view是否需要新建,否则使用缓存
Object data = getGroup(groupPosition);
从数据源中提取相应数据
TextView textView = view.findViewById(R.id.tv_group);
textView.setText(data.toString());
并为相应的TextView设置文本
if(groupPosition % 2 == 1){
textView.setTextColor(Color.RED);
}else{
textView.setTextColor(Color.BLUE);
}
为了看出自定义效果,在这里为文本设置颜色,其中数组位置为奇数的设置红色,偶数的设置蓝色。
子元素的设置类似父元素,在子元素中只是区分字体的大小,不赘述。
效果图:
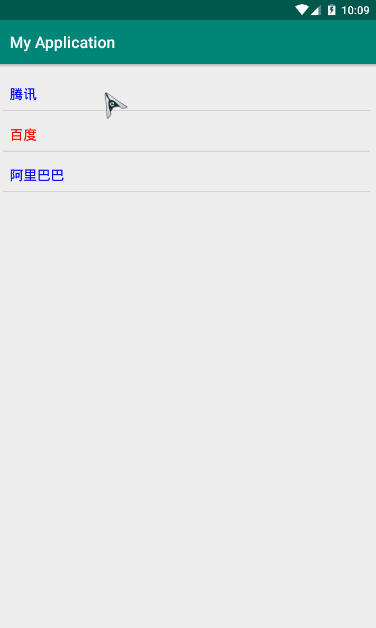
ExpandableListView之BaseExpandableListAdapter的更多相关文章
- 可展开的列表组件——ExpandableListView深入解析
可展开的列表组件--ExpandableListView深入解析 一.知识点 1.ExpandableListView常用XML属性 2.ExpandableListView继承BaseExpanda ...
- android适配器Adapter
一.什么是适配器,适配器有什么用? 适配器是AdapterView视图(如ListView - 列表视图控件.Gallery - 缩略图浏览器控件.GridView - 网格控件.Spinner - ...
- android 编程小技巧(持续中)
first: Intent跳转一般存用于Activity类,可是若要在非activity类里跳转的话,解决方法是在startActivity(intent)前加mContext即上下文,终于为 ...
- Android ExpandableListView BaseExpandableListAdapter (类似QQ分组列表)
分组列表视图(ExpandableListView) 和ListView不同的是它是一个两级的滚动列表视图,每一个组可以展开,显示一些子项,类似于QQ列表,这些项目来至于ExpandableListA ...
- Android开发之ExpandableListView扩展(BaseExpandableListAdapter的使用)(完整版)
Android开发之ExpandableListView扩展(BaseExpandableListAdapter的使用)(完整版)
- Android中使用ExpandableListView实现微信通讯录界面(完善仿微信APP)
之前的博文<Android中使用ExpandableListView实现好友分组>我简单介绍了使用ExpandableListView实现简单的好友分组功能,今天我们针对之前的所做的仿微信 ...
- Android中使用ExpandableListView实现好友分组
一个视图显示垂直滚动两级列表中的条目.这不同于列表视图,允许两个层次,类似于QQ的好友分组.要实现这个效果的整体思路为: 1.要给ExpandableListView 设置适配器,那么必须先设置数据源 ...
- 安卓开发树形控件之ExpandableListView(一)
这个例子非常简单,简单到一个初学者都能随便开发出来,今天的目的仅仅只是为了将效果实现出来,如果想深入这里有几篇非常不错的博客: Android 之ExpandableListView几个特殊的属性 h ...
- ExpandableListView实现展开更多和收起更多
[需求]: 如上面图示 当点开某个一级菜单的时候,其他菜单收起: 子级菜单默认最多5个: 多于5个的显示"展开更多" 点击"展开更多",展开该级所有子级菜单,同 ...
随机推荐
- C# 读取CAD文件缩略图(DWG文件)
//C# 读取CAD文件缩略图(DWG文件) https://blog.csdn.net/hanghangaidoudou/article/details/8589574 //2010-09-04 1 ...
- 【原创】微信小程序支付java后台案例(公众号支付同适用)(签名错误问题)
前言 1.微信小程序支付官方接口文档:[点击查看微信开放平台api开发文档]2.遇到的坑:预支付统一下单签名结果返回[签名错误]失败,建议用官方[签名验证工具]检查签名是否存在问题.3.遇到的坑:签名 ...
- 在一个jsp页面内实现简单计算器
首先创建一个calculate.jsp 这是用Javascript代码来验证,代码如下: <script type="text/javascript"> functio ...
- Zabbix-Web监控介绍篇
一.Web监控需求 监控一台Zabbix 3.0的WEB服务是否正常,包括登陆页,登陆后页面,退出页面 ps:zabbix的WEB监控可以实现登录后监控 二.监控环境介绍 监控服务器版本:zabbix ...
- Web Worker 使用教程
一.概述 JavaScript 语言采用的是单线程模型,也就是说,所有任务只能在一个线程上完成,一次只能做一件事.前面的任务没做完,后面的任务只能等着.随着电脑计算能力的增强,尤其是多核 CPU 的出 ...
- 随笔编号-07 JS针对时间操作
//获取完整的当前日期 var date=new Date; var year=date.getFullYear(); var month=date.getMonth()+1; month =(mon ...
- ConcurrentLinkedQueue 源码解读
一.介绍 ConcurrentLinkedQueue 是一个基于链接节点的无界线程安全队列,它采用先进先出的规则对节点进行排序,当我们添加一个元素的时候,它会添加到队列的尾部:当我们获取一个元素时,它 ...
- ssh三大框架的认识
一.SSH三大框架的概述 ssh为 struts+spring+hibernate的一个集成框架,是目前较流行的一种Web应用程序开源框架. 集成SSH框架的系统从职责上分为四层:表示层.业务逻辑层 ...
- 使用python发生邮箱
1.在使用邮箱登陆需要在邮箱内开启SMTP服务 2.注意在代码中登陆程序使用的密码为第三方授权登陆码,QQ邮箱为系统提供的授权码 网易邮箱为自己设置的授权码 QQ邮箱模拟 import smtplib ...
- bzoj 2002 弹飞绵羊 lct裸题
上一次用分块过了, 今天换了一种lct(link-cut tree)的写法. 学lct之前要先学过splay. lct 简单的来说就是 一颗树, 然后每次起作用的都是其中的某一条链. 所以每次如果需要 ...