求n的因子个数与其因子数之和
方法一:朴素算法:O(n)。
- #include<bits/stdc++.h>
- using namespace std;
- int get_num(int n){
- int num=;
- for(int i=;i<=n;++i)
- if(n%i==)num++;
- return num;
- }
- int get_sum(int n){
- int tot=;
- for(int i=;i<=n;++i)
- if(n%i==)tot+=i;
- return tot;
- }
- int main(){
- int n;
- while(cin>>n){
- cout<<get_num(n)<<endl;//求n的因子个数
- cout<<get_sum(n)<<endl;//求n的因子数之和
- }
- return ;
- }
方法二:约数个数定理:O(√n)。
- #include<bits/stdc++.h>
- using namespace std;
- int get_num(int n){
- int tot=;
- for(int i=;i*i<=n;++i){
- if(n%i==){
- int x=;
- while(n%i==){
- n/=i;
- x++;
- }
- tot*=(x+);
- }
- }
- if(n>)tot*=;
- return tot;
- }
- int get_sum(int n){
- int tot=;
- for(int i=;i*i<=n;i++){
- if(n%i==){
- int mul=;
- while(n%i==){
- n/=i;
- mul*=i;
- }
- tot*=(mul*i-)/(i-);
- }
- }
- if(n>)tot*=(n+);
- return tot;
- }
- int main(){
- int n;
- while(cin>>n){
- cout<<get_num(n)<<endl;//求n的因子个数
- cout<<get_sum(n)<<endl;//求n的因子数之和
- }
- return ;
- }
实战例题:
题解报告:hdu 2521 反素数
Problem Description
Input
输入包括a,b, 1<=a<=b<=5000,表示闭区间[a,b].
Output
Sample Input
2 3
1 10
47 359
Sample Output
2的因子为:1 2
- #include<bits/stdc++.h>
- using namespace std;
- int n,a,b,maxnum,maxindex;
- int get_num(int n){//求n的因子个数
- int tot=;
- for(int i=;i*i<=n;++i){
- if(n%i==){
- int x=;
- while(n%i==){
- n/=i;
- x++;
- }
- tot*=(x+);
- }
- }
- if(n>)tot*=;
- return tot;
- }
- int main(){
- while(cin>>n){
- while(n--){
- cin>>a>>b;maxnum=;maxindex=a;
- for(int i=a;i<=b;++i){
- int tmp=get_num(i);
- if(tmp>maxnum){maxindex=i;maxnum=tmp;}
- }
- cout<<maxindex<<endl;
- }
- }
- return ;
- }
题解报告:hdu 1215 七夕节
Problem Description
人们纷纷来到告示前,都想知道谁才是自己的另一半.告示如下:
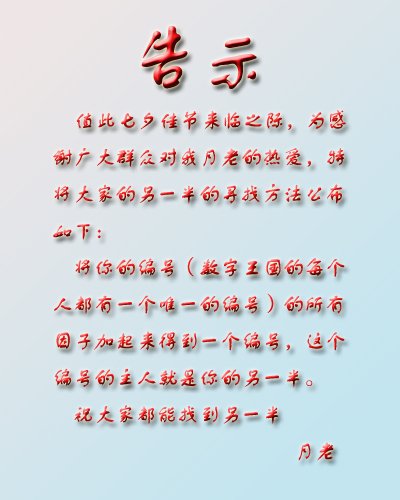
你想知道你的另一半吗?
Input
Output
Sample Input
Sample Output
- #include<bits/stdc++.h>
- using namespace std;
- int t,n;
- int get_sum(int n){
- int tot=;
- for(int i=;i*i<=n;++i){
- if(n%i==){
- int mul=;
- while(n%i==){
- n/=i;
- mul*=i;
- }
- tot*=(mul*i-)/(i-);
- }
- }
- if(n>)tot*=(n+);
- return tot;
- }
- int main(){
- while(~scanf("%d",&t)){
- while(t--){
- scanf("%d",&n);
- printf("%d\n",get_sum(n)-n);
- }
- }
- return ;
- }
AC代码二(109ms):简单打表,时间复杂度为nlogn。
- #include<bits/stdc++.h>
- const int maxn=;
- using namespace std;
- int t,n,sum[maxn];
- int main(){
- for(int i=;i<maxn/;++i)//因子i
- for(int j=i*;j<maxn;j+=i)//j是i的倍数,即j的因子是i
- sum[j]+=i;//sum[j]表示其所有因子之和
- while(~scanf("%d",&t)){
- while(t--){
- scanf("%d",&n);
- printf("%d\n",sum[n]);
- }
- }
- return ;
- }
题解报告:hdu 1999 不可摸数
Problem Description
Input
Output
Sample Input
Sample Output
- #include<bits/stdc++.h>
- const int maxn=;
- using namespace std;
- int t,n,sum[maxn];bool f[maxn];
- int main(){
- memset(f,false,sizeof(f));
- for(int i=;i<maxn/;++i)//因子i
- for(int j=i*;j<maxn;j+=i)//j是i的倍数,即j的因子是i
- sum[j]+=i;//sum[j]表示其所有因子之和
- for(int i=;i<maxn;++i)
- if(sum[i]<=)f[sum[i]]=true;//如果真因数之和在1000以内,那么sum[i]不是不可摸数
- while(~scanf("%d",&t)){
- while(t--){
- scanf("%d",&n);
- if(f[n])printf("no\n");
- else printf("yes\n");//n为不可摸数
- }
- }
- return ;
- }
AC代码二(0ms):
- #include<bits/stdc++.h>
- using namespace std;
- int t,n;bool f[];
- int main(){
- int obj[]={,,,,,,,,,,
- ,,,,,,,,,,,,
- ,,,,,,,,,,,,
- ,,,,,,,,,,,,
- ,,,,,,,,,,,,
- ,,,,,,,,,,,,
- ,,,,,,,,,,,,
- ,,,,,,};
- memset(f,false,sizeof(f));
- for(int i=;i<;++i)f[obj[i]]=true;//1000以内共有89个数为不可摸数
- while(~scanf("%d",&t)){
- while(t--){
- scanf("%d",&n);
- if(f[n])printf("yes\n");
- else printf("no\n");
- }
- }
- return ;
- }
题解报告:hdu 1299 Diophantus of Alexandria
Problem Description
Consider the following diophantine equation:
1 / x + 1 / y = 1 / n where x, y, n ∈ N+ (1)Diophantus is interested in the following question: for a given n, how many distinct solutions (i. e., solutions satisfying x ≤ y) does equation (1) have? For example, for n = 4, there are exactly three distinct solutions:
1 / 5 + 1 / 20 = 1 / 4
1 / 6 + 1 / 12 = 1 / 4
1 / 8 + 1 / 8 = 1 / 4Clearly, enumerating these solutions can become tedious for bigger values of n. Can you help Diophantus compute the number of distinct solutions for big values of n quickly?
Input
Output
Sample Input
Sample Output
3
113
- #include<bits/stdc++.h>
- using namespace std;
- typedef long long LL;int t;LL n;
- LL get_num(LL x){
- LL ans=,tp;
- for(LL i=;i*i<=x;++i){
- if(x%i==){
- tp=;
- while(x%i==)tp++,x/=i;
- ans*=(+*tp);
- }
- }
- if(x>)ans*=;
- return ans;
- }
- int main(){
- while(cin>>t){
- for(int i=;i<=t;++i){
- cin>>n;
- cout<<"Scenario #"<<i<<":\n"<<(get_num(n)+)/<<"\n"<<endl;
- }
- }
- return ;
- }
题解报告:NYOJ #66 分数拆分
描述
现在输入一个正整数k,找到所有的正整数x>=y,使得1/k=1/x+1/y.
输入
第一行输入一个整数n,代表有n组测试数据。
接下来n行每行输入一个正整数k
输出
按顺序输出对应每行的k找到所有满足条件1/k=1/x+1/y的组合。
样例输入
- 2
- 2
- 12
样例输出
- 1/2=1/6+1/3
- 1/2=1/4+1/4
- 1/12=1/156+1/13
- 1/12=1/84+1/14
- 1/12=1/60+1/15
- 1/12=1/48+1/16
- 1/12=1/36+1/18
- 1/12=1/30+1/20
- 1/12=1/28+1/21
- 1/12=1/24+1/24
解题思路:由x>=y且x、y均大于k可知1/x<=1/y,1/k-1/y<=1/y,即k<y<=2k,所以只需控制y的枚举范围即可。
AC代码:
- #include<bits/stdc++.h>
- using namespace std;
- int t,k;
- int main(){
- while(cin>>t){
- while(t--){
- cin>>k;
- for(int i=k+;i<=*k;++i)//枚举k+1~2k
- if(i*k%(i-k)==)printf("1/%d=1/%d+1/%d\n",k,i*k/(i-k),i);//通分,其中(i-k)|(i*k)。
- }
- }
- return ;
- }
财大情侣
Time Limit: 2000/1000ms (Java/Others)
Problem Description:
- 高考完终于来到梦寐以求的财经大学,男三女七有木有!食堂吃饭前后左右三排都是女生有木有!该学校总共有n个人,每个人都有对应自己的魅力值,从1到n。现规定每个人的情商为每个人魅力值的约数(不包括本身)之和。在广财有个很神奇的事情,当一个人的情商刚好等于另一个的魅力值的时候,这两个人就meant to be情侣(不是一男一女怎么办?随便吧)。
- 如:220:1+2+4+5+10+11+20+22+44+55+110=284
- 284:1+2+4+71+142=220
- 这样魅力值为220和284的就成为一对啦。
- 给定两个数,a,b,找出所有a和b之间(inclusive)成对的。
Input:
- 输入包含多组测试数据,每组数据输入两个数a,b (0<=a,b<=100000).
Output:
- 对于每组测试,输出a和b之间的所有情侣。每对情侣占一行,魅力值小的在前面。对于多对情侣,按情侣中魅力值小的排序。参考sample output。
Sample Input:
- 200 1300
- 1 200
Sample Output:
- 220 284
- 1184 1210
- NO
解题思路:求每个数的所有真因子之和,简单打个表再判断一下即可。
AC代码:
- #include<bits/stdc++.h>
- const int maxn=;//范围大一点,否则会出现越界的情况
- using namespace std;
- int a,b,x,sum[maxn];bool flag;
- int main(){
- for(int i=;i<maxn/;++i)//求因子数之和
- for(int j=i*;j<maxn;j+=i)//j初始为i的2倍,以后以i的步长增长,累加j的真因子
- sum[j]+=i;
- while(cin>>a>>b){
- if(a>b)swap(a,b);flag=false;
- for(int i=a;i<=b;++i)//先判断是不是一对情侣,并且前面的因子数之和x要在区间范围内,且前一个数i要小于后一个数x
- if(i==sum[x=sum[i]]&&x<=b&&i<x){flag=true;cout<<i<<' '<<x<<endl;}
- if(!flag)cout<<"NO"<<endl;
- }
- return ;
- }
求n的因子个数与其因子数之和的更多相关文章
- Soldier and Number Game---cf546D(打表求n的素因子个数)
题目链接:http://codeforces.com/problemset/problem/546/D 题意: 给出一个n,n开始是a!/b!,每次用一个x去整除n得到新的n,最后当n变成1的时候经过 ...
- Almost All Divisors(求因子个数及思维)
---恢复内容开始--- We guessed some integer number xx. You are given a list of almost all its divisors. Alm ...
- LightOj1028 - Trailing Zeroes (I)---求因子个数
题目链接:http://lightoj.com/volume_showproblem.php?problem=1028 题意:给你一个数 n (1<=n<=10^12), 然后我们可以把它 ...
- POJ 2992 Divisors (求因子个数)
题意:给n和k,求组合C(n,k)的因子个数. 这道题,若一开始先预处理出C[i][j]的大小,再按普通方法枚举2~sqrt(C[i][j])来求解对应的因子个数,会TLE.所以得用别的方法. 在说方 ...
- Acdream1084 寒假安排 求n!中v因子个数
题目链接:pid=1084">点击打开链接 寒假安排 Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 128000/64000 ...
- Trailing Zeroes (I) LightOJ - 1028(求因子个数)
题意: 给出一个N 求N有多少个别的进制的数有后导零 解析: 对于一个别的进制的数要转化为10进制 (我们暂且只分析二进制就好啦) An * 2^(n-1) + An-1 * 2^(n-2) + `` ...
- 第13届景驰-埃森哲杯广东工业大学ACM程序设计大赛-等式(求$N^2$的因子个数)
一.题目链接 https://www.nowcoder.com/acm/contest/90/F 二.题面 时间限制:C/C++ 1秒,其他语言2秒 空间限制:C/C++ 32768K,其他语言655 ...
- 求n!中因子k的个数
思路: 求n的阶乘某个因子k的个数,如果n比较小,可以直接算出来,但是如果n很大,此时n!超出了数据的表示范围,这种直接求的方法肯定行不通.其实n!可以表示成统一的方式. n!=(km)*(m!)*a ...
- BZOJ3994:约数个数和(莫比乌斯反演:求[1,N]*[1,M]的矩阵的因子个数)
Description 设d(x)为x的约数个数,给定N.M,求 Input 输入文件包含多组测试数据. 第一行,一个整数T,表示测试数据的组数. 接下来的T行,每行两个整数N.M. Outpu ...
随机推荐
- java面试题(摘录)
1.抽象,继承,封装,多态 2.基本数据类型的字节数 byte:1.int:4.char:2.long:8.float:4.double:8.boolean:1 和short:2 3.String , ...
- nlp_tool
http://www.afenxi.com/post/9700 11款开放中文分词引擎大比拼 附录评测数据地址http://bosonnlp.com/dev/resource 各家分词系统链接地址Bo ...
- IDEA中Git的应用场景
工作中多人使用版本控制软件协作开发,常见的应用场景归纳如下: 假设小组中有两个人,组长小张,组员小袁 场景一:小张创建项目并提交到远程Git仓库 场景二:小袁从远程git仓库上获取项目源码 场景三:小 ...
- luogu2398 SUM GCD
题目大意:求sum i(1->n) (sum j(1->n) (gcd(i,j))). 对于每对(i,j)都来一次gcd很慢,但是我们知道,一个约数i在1~n范围内是n/i个数的约数.gc ...
- VC FTP服务器程序分析(四)
下面是数据传输的重点-CDataSocket类,函数不多,都比较重要. 1.OnAccept 数据tcp服务器被连接的虚函数,由框架调用. void CDataSocket::OnAccept(in ...
- ssl原理及应用
今天学习网络通信,看到使用ssl(Secure Sockets Layer)进行加密,由于对ssl只是有些概念上的了解,对于具体应用原理.过程和如何使用不慎了解,于是学习了一番,总结如下: 1. 为什 ...
- Laravel中常见的错误与解决方法小结
一.报错: 「Can't swap PDO instance while within transaction」 通过查询 Laravel 源代码,可以确认异常是在 setPdo 方法中抛出的: ? ...
- vue-resourse 提交表单 使用formData
通过formData对象可以组装一组用XMLHttpRequest发送请求的键/值对.它可以更灵活方便的发送表单数据,因为可以独立于表单使用.如果把表单的编码类型设置为multipart/form-d ...
- -webkit-text-size-adjust 处理设置字体<12px
-webkit-text-size-adjust 1.当样式表里font-size<12px时,中文版chrome浏览器里字体显示仍为12px,这时可以用 html{-webkit-text- ...
- Python 赋值、浅拷贝和深拷贝
初学Python,和C++还是有许多不同.直接赋值.浅拷贝和深拷贝,这三种拷贝对象的操作之间还是有许多的区别.Python语言的版本为2.7,在Pycharm中进行实验. 一.直接赋值 用下面的代码来 ...