Power Network (最大流增广路算法模板题)
Time Limit: 2000MS | Memory Limit: 32768K | |
Total Submissions: 20754 | Accepted: 10872 |
Description
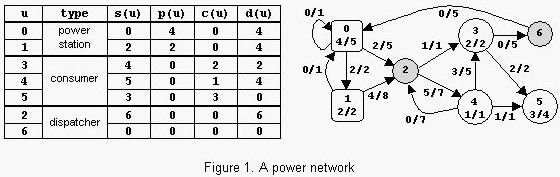
An example is in figure 1. The label x/y of power station u shows that p(u)=x and pmax(u)=y. The label x/y of consumer u shows that c(u)=x and cmax(u)=y. The label x/y of power transport line (u,v) shows that l(u,v)=x and lmax(u,v)=y. The power consumed is Con=6. Notice that there are other possible states of the network but the value of Con cannot exceed 6.
Input
Output
Sample Input
2 1 1 2 (0,1)20 (1,0)10 (0)15 (1)20
7 2 3 13 (0,0)1 (0,1)2 (0,2)5 (1,0)1 (1,2)8 (2,3)1 (2,4)7
(3,5)2 (3,6)5 (4,2)7 (4,3)5 (4,5)1 (6,0)5
(0)5 (1)2 (3)2 (4)1 (5)4
Sample Output
15
6
Hint
#include<stdio.h>
#include<string.h>
#include<queue>
using namespace std; const int MAX = ;
const int INF = 0x3f3f3f3f;
int n,np,nc,m,mf,s,t;
int cap[MAX][MAX],flow[MAX][MAX],a[MAX];
int pre[MAX];
char str[];
queue <int> que;
void maxflow()
{
memset(flow,,sizeof(flow));//初始化,所有的边的流量初始为0;
mf = ;//记录最大流
for(;;)
{
memset(a,,sizeof(a));//s到每个节点路径上的最小残量
a[s] = INF;
que.push(s);
//bfs找增广路
while(!que.empty())
{
int u = que.front();
que.pop();
for(int v = ; v <= n+; v++)
{
if(!a[v] && cap[u][v] > flow[u][v])//找到新的节点v
{
pre[v] = u;//记录前驱并加入队列
que.push(v);
if(a[u] < cap[u][v]-flow[u][v])
a[v] = a[u];
else a[v] = cap[u][v]-flow[u][v];//s到v路径上的最小残量
}
}
}
if(a[t] == ) break;//找不到最小残量,当前流已经是最大流;
for(int u = t; u!= s;u = pre[u])//从汇点往回走
{
flow[pre[u]][u] += a[t];//更新正向流量
flow[u][pre[u]] -= a[t];//更新反向流量
}
mf += a[t];//更新从s流出的总流量
}
} int main()
{
int u,v,z;
while(~scanf("%d %d %d %d",&n,&np,&nc,&m))
{
memset(cap,,sizeof(cap));
while(m--)
{
scanf("%s",str);
sscanf(str,"(%d,%d)%d",&u,&v,&z);
cap[u][v] = z;
} while(np--)//有多个起点
{
scanf("%s",str);
sscanf(str,"(%d)%d",&v,&z);
cap[n][v] = z;//将多个起点连接到一个新的顶点作为起点;
} while(nc--)//有多个终点
{
scanf("%s",str);
sscanf(str,"(%d)%d",&u,&z);
cap[u][n+] = z;//将多个终点连接到一个新的终点作为终点;
}
s = n;
t = n+;
maxflow();
printf("%d\n",mf);
}
return ;
}
Power Network (最大流增广路算法模板题)的更多相关文章
- hdu 3549 Flow Problem【最大流增广路入门模板题】
题目:http://acm.hdu.edu.cn/showproblem.php?pid=3549 Flow Problem Time Limit: 5000/5000 MS (Java/Others ...
- HDU3549 Flow Problem(网络流增广路算法)
题目链接. 分析: 网络流增广路算法模板题.http://www.cnblogs.com/tanhehe/p/3234248.html AC代码: #include <iostream> ...
- 网络流初步:<最大流>——核心(增广路算法)(模板)
增广路的核心就是引入了反向边,使在进行道路探索选择的时候增加了类似于退路的东西[有一点dp的味道??] 具体操作就是:1.首先使用结构体以及数组链表next[ MAXN ]进行边信息的存储 2.[核心 ...
- hdu 3549 Flow Problem(增广路算法)
题目:http://acm.hdu.edu.cn/showproblem.php?pid=3549 模板题,白书上的代码... #include <iostream> #include & ...
- 最大流增广路(KM算法) HDOJ 2255 奔小康赚大钱
题目传送门 /* KM:裸题第一道,好像就是hungary的升级版,不好理解,写点注释 KM算法用来解决最大权匹配问题: 在一个二分图内,左顶点为X,右顶点为Y,现对于每组左右连接Xi,Yj有权w(i ...
- 最大流增广路(KM算法) HDOJ 1533 Going Home
题目传送门 /* 最小费用流:KM算法是求最大流,只要w = -w就可以了,很经典的方法 */ #include <cstdio> #include <cmath> #incl ...
- 最大流增广路(KM算法) HDOJ 1853 Cyclic Tour
题目传送门 /* KM: 相比HDOJ_1533,多了重边的处理,还有完美匹配的判定方法 */ #include <cstdio> #include <cmath> #incl ...
- 网络最大流增广路模板(EK & Dinic)
EK算法: int fir[maxn]; int u[maxm],v[maxm],cap[maxm],flow[maxm],nex[maxm]; int e_max; int p[maxn],q[ma ...
- 网络流——增广路算法(dinic)模板 [BeiJing2006]狼抓兔子
#include<iostream> #include<cstring> #include<algorithm> #include<cmath> #in ...
随机推荐
- 最蛋疼的bug:读取图片缩略图(一定要在相冊查看下形成缓存)
近期的一个连接服务端的应用.须要读取图片,一般供用户公布商品选择上传图片.初始的图片列表应该是缩略图.仅仅有确定了,才上传原图,OK不多说上代码 package edu.buaa.erhuo; imp ...
- 几种任务调度的 Java 实现方法与比较--转载
前言 任务调度是指基于给定时间点,给定时间间隔或者给定执行次数自动执行任务.本文由浅入深介绍四种任务调度的 Java 实现: Timer ScheduledExecutor 开源工具包 Quartz ...
- iOS---多线程实现方案一 (pthread、NSThread)
在iOS开发中,多线程是我们在开发中经常使用的一门技术.那么本文章将和大家探讨一下针对于多线程的技术实现.本文主要分为如下几个部分: iOS开发中实现多线程的方式 单线程 pthread NSThre ...
- readonly 与 const
readonly MSDN定义:readonly 关键字是可以在字段上使用的修饰符.当字段声明包括 readonly 修饰符时,该声明引入的字段赋值只能作为声明的一部分出现,或者出现在同一类的构造函数 ...
- Ecstore后台中显示页面display,page,singlepage方法的区别?
dispaly 显示的页面不包含框架的其他页面,只是本身的页面(使用范围:Ecstore的前端.后端). page 显示的页面包含在框架的里面(使用范围:Ecstore的前端.后端). singlep ...
- asp.net web.config的学习笔记
原文地址:http://www.cnblogs.com/Bulid-For-NET/archive/2013/01/11/2856632.html 一直都对web.config不太清楚.这几天趁着项目 ...
- 把某个asp.net 控件 替换成 自定义的控件
功能:可以把某个asp.net 控件 替换成 自定义的控件 pages 的 tagMapping 元素(ASP.NET 设置架构) 定义一个标记类型的集合,这些标记类型在编译时重新映射为其他标记类型. ...
- (转)PHP函数spl_autoload_register()用法和__autoload()介绍
转--http://www.jb51.net/article/29624.htm 又是框架冲突导致__autoload()失效,用spl_autoload_register()重构一下,问题解决 ...
- (转)织梦DedeCMSv5.7安装体验数据包的方法
1.先安装一个全新的DedeCMS v5.7 GBK系统,这里以DedeCMS v5.7 GBK系统为例. 2.下载数据体验包: 如果是gbk则下载:http://www.dedecms.com/de ...
- Objective-C基础知识点总结
一.#import 和 #include 的区别,@class代表什么?@class 和 #import 的区别?#import<> 和 #import""的区别 答: ...