高效算法——B 抄书 copying books,uva714
Description
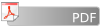
Before the invention of book-printing, it was very hard to make a copy of a book. All the contents had to be re-written by hand by so calledscribers. The scriber had been given a book and after several months he finished its copy. One of the most famous scribers lived in the 15th century and his name was Xaverius Endricus Remius Ontius Xendrianus (Xerox). Anyway, the work was very annoying and boring. And the only way to speed it up was to hire more scribers.
Once upon a time, there was a theater ensemble that wanted to play famous Antique Tragedies. The scripts of these plays were divided into many books and actors needed more copies of them, of course. So they hired many scribers to make copies of these books. Imagine you have m books (numbered ) that may have different number of pages (
) and you want to make one copy of each of them. Your task is to divide these books among k scribes,
. Each book can be assigned to a single scriber only, and every scriber must get a continuous sequence of books. That means, there exists an increasing succession of numbers
such that i-th scriber gets a sequence of books with numbers between bi-1+1 and bi. The time needed to make a copy of all the books is determined by the scriber who was assigned the most work. Therefore, our goal is to minimize the maximum number of pages assigned to a single scriber. Your task is to find the optimal assignment.
Input
The input consists of N cases. The first line of the input contains only positive integer N. Then follow the cases. Each case consists of exactly two lines. At the first line, there are two integers m and k, . At the second line, there are integers
separated by spaces. All these values are positive and less than 10000000.
Output
For each case, print exactly one line. The line must contain the input succession divided into exactly k parts such that the maximum sum of a single part should be as small as possible. Use the slash character (`/') to separate the parts. There must be exactly one space character between any two successive numbers and between the number and the slash.
If there is more than one solution, print the one that minimizes the work assigned to the first scriber, then to the second scriber etc. But each scriber must be assigned at least one book.
Sample Input
2
9 3
100 200 300 400 500 600 700 800 900
5 4
100 100 100 100 100
Sample Output
100 200 300 400 500 / 600 700 / 800 900
100 / 100 / 100 / 100 100
解题思路:
题意:按顺序给你N个数,将这N个数分成连续的M段,使得这M段每段的和中的最大值最小,输出最小值(1<=N<=100000,1<=M<=N,每个数在1到10000之间),如果有多种可能的话,尽量在前面进行划分。
思路:
1、由于函数具有单调性的特征,因此可以用二分枚举的办法去实现它,但这里不需要排序。
2、输出的时候需要用到贪心的思想,既尽量往前划分。
3、大概的思路就是二分枚举求得满足题意的最大值之后,然后以这个最大值通过从后往前的方式划分成段,如果剩余可划分段与i+1的值相等(尽量靠前),则将剩余的段往前划分,具体实现可以用一个标记数组表示是否划分。
5、注意要用long long 来存。
解题思路借鉴了大神的,感觉这个思路比较清晰、易懂,希望能够帮助到博友们
程序代码:
#include<cstdio>
#include<cstring>
#include<algorithm>
using namespace std;
const int maxm = + ;
int m, k, p[maxm];
int solve(long long maxp)
{
long long done = ;
int ans = ;
for(int i = ; i < m; i++)
{
if(done + p[i] <= maxp) done += p[i];
else { ans++; done = p[i]; }
}
return ans;
}
int last[maxm];
void print(long long ans)
{
long long done = ;
memset(last, , sizeof(last));
int remain = k;
for(int i = m-; i >= ; i--)
{
if(done + p[i] > ans || i+ < remain)
{ last[i] = ; remain--; done = p[i]; }
else
done += p[i];
}
for(int i = ; i < m-; i++)
{
printf("%d ", p[i]);
if(last[i]) printf("/ ");
}
printf("%d\n", p[m-]);
}
int main()
{
int T;
scanf("%d", &T);
while(T--)
{
scanf("%d%d", &m, &k);
long long tot = ;
int maxp = -;
for(int i = ; i < m; i++)
{
scanf("%d", &p[i]);
tot += p[i];
maxp = max(maxp, p[i]);
}
long long L = maxp, R = tot;
while(L < R)
{
long long M = L + (R-L)/;
if(solve(M) <= k) R = M; else L = M+;
}
print(L);
}
return ;
}
高效算法——B 抄书 copying books,uva714的更多相关文章
- 抄书 Copying Books UVa 714
Copying Books 题目链接:http://acm.hust.edu.cn/vjudge/contest/view.action?cid=85904#problem/B 题目: Descri ...
- POJ1505&&UVa714 Copying Books(DP)
Copying Books Time Limit: 3000MS Memory Limit: 10000K Total Submissions: 7109 Accepted: 2221 Descrip ...
- UVa 714 Copying Books(二分)
题目链接: 传送门 Copying Books Time Limit: 3000MS Memory Limit: 32768 KB Description Before the inventi ...
- UVA 714 Copying Books 二分
题目链接: 题目 Copying Books Time limit: 3.000 seconds 问题描述 Before the invention of book-printing, it was ...
- poj 1505 Copying Books
http://poj.org/problem?id=1505 Copying Books Time Limit: 3000MS Memory Limit: 10000K Total Submiss ...
- 深入N皇后问题的两个最高效算法的详解 分类: C/C++ 2014-11-08 17:22 117人阅读 评论(0) 收藏
N皇后问题是一个经典的问题,在一个N*N的棋盘上放置N个皇后,每行一个并使其不能互相攻击(同一行.同一列.同一斜线上的皇后都会自动攻击). 一. 求解N皇后问题是算法中回溯法应用的一个经典案例 回溯算 ...
- uva 714 Copying Books(二分法求最大值最小化)
题目连接:714 - Copying Books 题目大意:将一个个数为n的序列分割成m份,要求这m份中的每份中值(该份中的元素和)最大值最小, 输出切割方式,有多种情况输出使得越前面越小的情况. 解 ...
- UVA 714 Copying Books 最大值最小化问题 (贪心 + 二分)
Copying Books Before the invention of book-printing, it was very hard to make a copy of a book. A ...
- Copying Books
Copying Books 给出一个长度为m的序列\(\{a_i\}\),将其划分成k个区间,求区间和的最大值的最小值对应的方案,多种方案,则按从左到右的区间长度尽可能小(也就是从左到右区间长度构成的 ...
随机推荐
- css3购物网站商品文字提示实例
css3购物网站商品文字提示实例先来看效果图:<ignore_js_op> 当鼠标划过图片时,有着泰迪熊黑色长方形的背景就会出现.来看HTML5+CSS3代码: <!DOCTYPE ...
- Mysql表复制及备份还原
1.复制表结构 1.1 含有主键等信息的完整表结构 CREATE table 新表名 LIKE book; 1.2 只有表结构,没有主键等信息 create table 新表名 s ...
- Android之获取本地图片并压缩方法
这两天在做项目时,做到上传图片功能一块时,碰到两个问题,一个是如何获取所选图片的路径,一个是如何压缩图片,在查了一些资料和看了别人写的后总算折腾出来了,在此记录一下. 首先既然要选择图片,我们就先要获 ...
- Android App优化建议(转载)
假如要Google Play上做一个最失败的案例,那最好的秘诀就是界面奇慢无比.耗电.耗内存.接下来就会得到用户的消极评论,最后名声也就臭了.即使你的应用设计精良.创意无限也没用. 耗电或者内存占用等 ...
- 使用charles proxy for Mac来抓取手机App的网络包
之前做Web项目的时候,经常会使用Fiddler(Windows下).Charles Proxy(Mac下)来抓包,调试一些东西:现在搞Android App开发,有时候也需要分析手机App的网络请求 ...
- Java线程(学习整理)--2---加入另一个线程join
1.join简介: 今天刚学的,这里我简单总结一下,join本身就是“加入”的意思,那么在线程中是什么意思呢?是在一个线程的run方法执行过程中,当特殊情况下需要执行一些其他的操作的时候,我们会用到j ...
- nextDay、beforeDay以及根据nextDay(beforeDay)求解几天后的日期,几天前的日期和两个日期之间的天数
实现代码: package com.corejava.chap02; public class Date { private int year; private int month; private ...
- helloServlet
创建第一个web程序 用myeclipse创建一个web项目,继而创建一个servlet 自动帮助你创建了一个web.xml文件 <servlet>....<servlet>指 ...
- javascript——集合类
/** * Created by Administrator on 2015/4/14. */ function Set() { this.values = {}; this.n = 0; this. ...
- php开发利器
phpstorm 当前版本2016.1 之前用的为Zend studio,比之notepad++确实方便很多,不过很多方面还是不方便的,比如定位文件,上传下载到svn什么的. 看到phpstorm新版 ...