poj 2299 Ultra-QuickSort :归并排序求逆序数
Time Limit: 7000MS | Memory Limit: 65536K | |
Total Submissions: 34676 | Accepted: 12465 |
Description
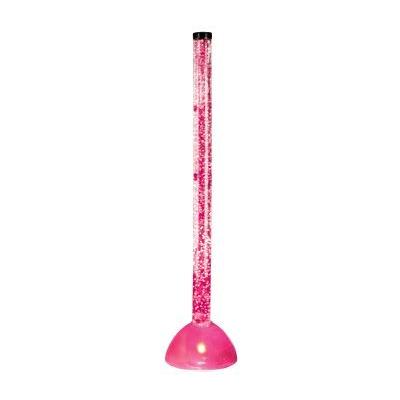
sorted in ascending order. For the input sequence
9 1 0 5 4 ,
Ultra-QuickSort produces the output
0 1 4 5 9 .
Your task is to determine how many swap operations Ultra-QuickSort needs to perform in order to sort a given input sequence.
Input
element. Input is terminated by a sequence of length n = 0. This sequence must not be processed.
Output
Sample Input
5
9
1
0
5
4
3
1
2
3
0
Sample Output
6
0
题目意图很明确了,就是给你一些数,求逆序数,但是数据量很大,普通的n^2求逆序数的方法铁定超时,所以只能用归并排序求逆序数,合并的时候,我们假设两部分为part1和part2,(part1在前,part2在后)这两部分已经排好序了,那么合并part1和part2的时候,如果part1的top1位置的数大于part2的top2位置的数,那么说明part1后面的那些数也都要比part2的top2位置的数大,所以逆序数就是mid到part1位置的距离
#include<stdio.h>
#include<iostream>
using namespace std; int array[5000001];
__int64 flag = 0; void merg(int head, int tail)
{
int mid = (tail + head) / 2 + 1;
int * new_array = new int[(tail - head) + 1];
int top1 = head;
int top2 = mid;
int i;
for(i = 0; top1 < mid && top2 <= tail ; i++)
{
if(array[top1] > array[top2])
{
new_array[i] = array[top2];
top2 ++;
}
else
{
new_array[i] = array[top1];
flag += top2 - (mid);
top1 ++;
}
}
if(top1 == mid && top2 <= tail)
{
while(top2 <= tail)
new_array[i++] = array[top2++];
}
else if(top1 != mid && top2 > tail)
{
while(top1 < mid)
{
new_array[i++] = array[top1++];
flag += tail - (mid) + 1;
}
}
memcpy(&array[head], new_array, sizeof(int) * (tail - head + 1) );
}
void mergsort(int head, int tail)
{
if(head >= tail)
return ;
mergsort(head, (head + tail) / 2);
mergsort((head + tail) / 2 + 1, tail);
merg(head, tail);
}
int main()
{
int n;
while(scanf("%d", &n), n != 0)
{
int i;
flag = 0;
for(i = 0; i < n; i++)
scanf("%d", &array[i]); mergsort(0, n - 1);
printf("%I64d\n", flag); }
return 0;
}
poj 2299 Ultra-QuickSort :归并排序求逆序数的更多相关文章
- 题解报告:poj 2299 Ultra-QuickSort(BIT求逆序数)
Description In this problem, you have to analyze a particular sorting algorithm. The algorithm proce ...
- poj 2299 树状数组求逆序数+离散化
http://poj.org/problem?id=2299 最初做离散化的时候没太确定可是写完发现对的---由于后缀数组学的时候,,这样的思维习惯了吧 1.初始化as[i]=i:对as数组依照num ...
- POJ 2299 -Ultra-QuickSort-树状数组求逆序数
POJ 2299Ultra-QuickSort 使用树状数组记录逆序对数. 把数组按照大小顺序插入,getsum(i)就是i前面的比他大的数. #include <cstdio> #inc ...
- poj 2299 Ultra-QuickSort 归并排序求逆序数对
题目链接: http://poj.org/problem?id=2299 题目描述: 给一个有n(n<=500000)个数的杂乱序列,问:如果用冒泡排序,把这n个数排成升序,需要交换几次? 解题 ...
- [CF 351B]Jeff and Furik[归并排序求逆序数]
题意: 两人游戏, J先走. 给出一个1~n的排列, J选择一对相邻数[题意!!~囧], 交换. F接着走, 扔一硬币, 若正面朝上, 随机选择一对降序排列的相邻数, 交换. 若反面朝上, 随机选择一 ...
- POJ2299 Ultra-QuickSort(归并排序求逆序数)
归并排序求逆序数 Time Limit:7000MS Memory Limit:65536KB 64bit IO Format:%I64d & %I64u Descri ...
- HDU 3743 Frosh Week(归并排序求逆序数)
归并排序求逆序数 #include <iostream> #include <cstdio> using namespace std; #define maxn 1000005 ...
- hiho一下 第三十九周 归并排序求逆序数
题目链接:http://hihocoder.com/contest/hiho39/problem/1 ,归并排序求逆序数. 其实这道题也是可以用树状数组来做的,不过数据都比较大,所以要离散化预处理一下 ...
- poj 2299 Ultra-QuickSort (归并排序 求逆序数)
题目:http://poj.org/problem?id=2299 这个题目实际就是求逆序数,注意 long long 上白书上的模板 #include <iostream> #inclu ...
随机推荐
- 最长公共字串(LCS)最长连续公共字串(LCCS)
链接1:http://blog.csdn.net/x_xiaoge/article/details/7376220 链接2:http://blog.csdn.net/x_xiaoge/article/ ...
- mybatis 中的稍微复杂些的sql语句
mybatis 中的稍微复杂些的sql语句: <?xml version="1.0" encoding="UTF-8" ?> <!DOCTYP ...
- RMAN备份与恢复之删除过期备份
使用crosscheck backupset或crosscheck backup之后,提示所有备份集都为available状态,当他执行delete obsolete时,提示有两个文件需要删除.实际上 ...
- (转)【Android测试工具】03. ApkTool在Mac上的安装和使用(2.0版本)
http://blog.csdn.net/wirelessqa/article/details/8997168 http://code.google.com/p/android-apktool/dow ...
- MS CRM 2011的自定义和开发(11)——插件(plugin)开发(二)
http://www.cnblogs.com/StoneGarden/archive/2012/02/06/2339490.html MS CRM 2011的自定义和开发(11)——插件(plugin ...
- Linux下dig命令使用
Dig简介: Dig是一个在类Unix命令行模式下查询DNS包括NS记录,A记录,MX记录等相关信息的工具.由于一直缺失Dig man page文档,本文就权当一个dig使用向导吧. Dig的 ...
- Ubuntu Server上的LVM配置
在安装Linux的时候,通常遇到的一个比较头痛的问题就是分区,到底每个区该分多少,用了一段时间之后,某个分区又不够用了,该怎么办?如果是普通的服务器,那一切都好说,大不了就关机重新划分分区嘛,但是对于 ...
- angularjs中ng-route和ui-router简单用法的代码比较
1.使用ng-route: app.js中的写法: var app=angular.module('birthdayApp',['ngRoute']); app.config(function($ro ...
- 黄聪:如何用Jquery或者插件解除网页禁用右键复制的限制(转)
1.随便打开一个网址,放到收藏夹中. 2.复制下面的代码,替换原来网址的URL 选中复制以下代码 javascript:(function(){var doc=document;var bd=doc. ...
- 2. redis的数据类型
一. string类型 字符串类型是redis中最基本的数据类型,它能存储任何形式的内容,包含二进制数据,甚至是一张图片(二进制内容).一个字符串类型的值存储的最大容量是1GB 命令 (1)setnx ...