poj 1279 半平面交核面积
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 6668 | Accepted: 2725 |
Description
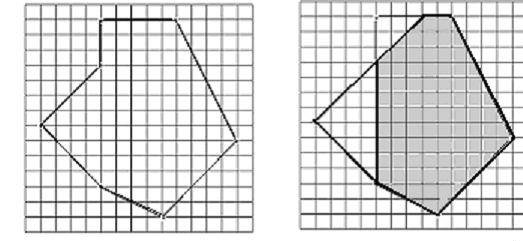
Input
Output
Sample Input
1
7
0 0
4 4
4 7
9 7
13 -1
8 -6
4 -4
Sample Output
80.00
/*
poj 1279 半平面交核面积 给你一个多边形的图书馆.要求得到一块地方能看见墙上所有的点,并求出面积
在半平面模板上加个求面积公式即可.
而且输入并没有指定顺时针还是逆时针,可以通过求面积进行判断. hhh-2016-05-11 21:01:47
*/
#include <iostream>
#include <vector>
#include <cstring>
#include <string>
#include <cstdio>
#include <queue>
#include <cmath>
#include <algorithm>
#include <functional>
#include <map>
using namespace std;
#define lson (i<<1)
#define rson ((i<<1)|1)
typedef long long ll;
using namespace std;
const int maxn = 1510;
const double PI = 3.1415926;
const double eps = 1e-8; int sgn(double x)
{
if(fabs(x) < eps) return 0;
if(x < 0)
return -1;
else
return 1;
} struct Point
{
double x,y;
Point() {}
Point(double _x,double _y)
{
x = _x,y = _y;
}
Point operator -(const Point &b)const
{
return Point(x-b.x,y-b.y);
}
double operator ^(const Point &b)const
{
return x*b.y-y*b.x;
}
double operator *(const Point &b)const
{
return x*b.x + y*b.y;
}
}; struct Line
{
Point s,t;
double k;
Line() {}
Line(Point _s,Point _t)
{
s = _s;
t = _t;
k = atan2(t.y-s.y,t.x-s.x);
}
Point operator &(const Line &b) const
{
Point res = s;
double ta = ((s-b.s)^(b.s-b.t))/((s-t)^(b.s-b.t));
res.x += (t.x-s.x)*ta;
res.y += (t.y-s.y)*ta;
return res;
}
}; bool HPIcmp(Line a,Line b)
{
if(fabs(a.k-b.k) > eps) return a.k<b.k;
return ((a.s-b.s)^(b.t-b.s)) < 0;
}
Line li[maxn]; double CalArea(Point p[],int n)
{
double ans = 0;
for(int i = 0;i < n;i++)
{
ans += (p[i]^p[(i+1)%n])/2;
}
return ans;
} double HPI(Line line[],int n,Point res[],int &resn)
{
int tot =n;
sort(line,line+n,HPIcmp);
tot = 1;
for(int i = 1; i < n; i++)
{
if(fabs(line[i].k - line[i-1].k) > eps)
line[tot++] = line[i];
}
int head = 0,tail = 1;
li[0] = line[0];
li[1] = line[1];
resn = 0;
for(int i = 2; i < tot; i++)
{
if(fabs((li[tail].t-li[tail].s)^(li[tail-1].t-li[tail-1].s)) < eps||
fabs((li[head].t-li[head].s)^(li[head+1].t-li[head+1].s)) < eps)
return 0;
while(head < tail && (((li[tail] & li[tail-1]) - line[i].s) ^ (line[i].t-line[i].s)) > eps)
tail--;
while(head < tail && (((li[head] & li[head+1]) - line[i].s) ^ (line[i].t-line[i].s)) > eps)
head++;
li[++tail] = line[i];
}
while(head < tail && (((li[tail] & li[tail-1]) - li[head].s) ^ (li[head].t-li[head].s)) > eps)
tail--;
while(head < tail && (((li[head] & li[head-1]) - li[tail].s) ^ (li[tail].t-li[tail].t)) > eps)
head++;
if(tail <= head+1)
return 0;
for(int i = head; i < tail; i++)
res[resn++] = li[i]&li[i+1];
if(head < tail-1)
res[resn++] = li[head]&li[tail]; double tans = 0;
for(int i = 0;i < resn;i++)
{
tans += (res[i]^res[(i+1)%resn])/2;
}
return fabs(tans);
}
Point p0;
Point lis[maxn];
Line line[maxn];
double dist(Point a,Point b)
{
return sqrt((a-b)*(a-b));
} bool cmp(Point a,Point b)
{
double t = (a-p0)^(b-p0);
if(sgn(t) > 0)return true;
else if(sgn(t) == 0 && sgn(dist(a,lis[0])-dist(b,lis[0])) <= 0)
return true;
else
return false;
} int main()
{
//freopen("in.txt","r",stdin);
int n,T;
scanf("%d",&T);
while(T--)
{
scanf("%d",&n);
for(int i = 0; i < n; i++)
{
scanf("%lf%lf",&lis[i].x,&lis[i].y);
}
int ans;
if(CalArea(lis,n) < 0)
reverse(lis,lis+n);
for(int i = 0; i < n; i++)
{
line[i] = Line(lis[i],lis[(i+1)%n]);
}
printf("%.2f\n",HPI(line,n,lis,ans));
}
return 0;
}
poj 1279 半平面交核面积的更多相关文章
- poj 1755 半平面交+不等式
Triathlon Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 6461 Accepted: 1643 Descrip ...
- poj 3335 /poj 3130/ poj 1474 半平面交 判断核是否存在 / poj1279 半平面交 求核的面积
/*************** poj 3335 点序顺时针 ***************/ #include <iostream> #include <cmath> #i ...
- poj 3525 半平面交求多边形内切圆最大半径【半平面交】+【二分】
<题目链接> 题目大意:给出一个四面环海的凸多边形岛屿,求出这个岛屿中的点到海的最远距离. 解题分析: 仔细思考就会发现,其实题目其实就是让我们求该凸多边形内内切圆的最大半径是多少.但是, ...
- POJ 3525 /// 半平面交 模板
题目大意: 给定n,接下来n行逆时针给定小岛的n个顶点 输出岛内离海最远的点与海的距离 半平面交模板题 将整个小岛视为由许多半平面围成 那么以相同的比例缩小这些半平面 一直到缩小到一个点时 那个点就是 ...
- POJ 3525 半平面交+二分
二分所能形成圆的最大距离,然后将每一条边都向内推进这个距离,最后所有边组合在一起判断时候存在内部点 #include <cstdio> #include <cstring> # ...
- POJ 3335 Rotating Scoreboard 半平面交求核
LINK 题意:给出一个多边形,求是否存在核. 思路:比较裸的题,要注意的是求系数和交点时的x和y坐标不要搞混...判断核的顶点数是否大于1就行了 /** @Date : 2017-07-20 19: ...
- poj 1271 && uva 10117 Nice Milk (半平面交)
uva.onlinejudge.org/index.php?option=com_onlinejudge&Itemid=8&page=show_problem&problem= ...
- poj 2451 Uyuw's Concert (半平面交)
2451 -- Uyuw's Concert 继续半平面交,这还是简单的半平面交求面积,不过输入用cin超时了一次. 代码如下: #include <cstdio> #include &l ...
- bzoj 2618 半平面交模板+学习笔记
题目大意 给你n个凸多边形,求多边形的交的面积 分析 题意\(=\)给你一堆边,让你求半平面交的面积 做法 半平面交模板 1.定义半平面为向量的左侧 2.将所有向量的起点放到一个中心,以中心参照进行逆 ...
随机推荐
- js日常积累
1.数组转字符串 str.join(',') 2.字符串转数组 arr.split(',') 3.数组排序 function sorb(a,b){return a-b;}; arr.sort(sorb ...
- 【iOS】swift-ObjectC 在iOS 8中使用UIAlertController
iOS 8的新特性之一就是让接口更有适应性.更灵活,因此许多视图控制器的实现方式发生了巨大的变化.全新的UIPresentationController在实现视图控制器间的过渡动画效果和自适应设备尺寸 ...
- Hibernate实体类注解解释
Hibernate注解1.@Entity(name="EntityName")必须,name为可选,对应数据库中一的个表2.@Table(name="",cat ...
- STM32F4系列单片机上使用CUBE配置MBEDTLS实现pem格式公钥导入
|版权声明:本文为博主原创文章,未经博主允许不得转载. 最近尝试在STM32F4下用MBEDTLS实现了公钥导入(我使用的是ECC加密),整个过程使用起来比较简单. 首先,STM32F4系列CUBE里 ...
- 【Fungus入门】10分钟快速构建Unity中的万能对话系统 / 叙事系统 / 剧情系统
我真的很久没有写过一个完整的攻略了(笑),咸鱼了很久之后还是想来写一个好玩的.这次主要是梳理一下Unity的小众插件Fungus的核心功能,并且快速掌握其使用方法. 官方文档:http://fungu ...
- eclipse maven项目目录
今天遇见一个错误,关于eclipse项目的路径问题,web-inf的路径,上图和下图出现了两种web-INF,src的web-INFf和webContent的web-INF,src里面的文件需要编译以 ...
- python subprocess模块使用总结
一.subprocess以及常用的封装函数运行python的时候,我们都是在创建并运行一个进程.像Linux进程那样,一个进程可以fork一个子进程,并让这个子进程exec另外一个程序.在Python ...
- 喜马拉雅音频下载工具 - xmlyfetcher
xmlyfetcher用于下载喜马拉雅歌曲资源,可以下载单个音频资源,也可以下载整个专辑. 项目地址:https://github.com/smallmuou/xmlyfetcher 安装 安装jsh ...
- 从零开始:一个正式的vue+webpack项目的目录结构是怎么形成的
如何从零开始一个vue+webpack前端工程工作流的搭建,首先我们先从项目的目录结构入手.一个持续可发展,不断加入新功能,方便后期维护的目录结构究竟是长什么样子的?接下来闰土大叔带你们一起手摸手学起 ...
- python Django之文件上传
python Django之文件上传 使用Django框架进行文件上传共分为俩种方式 一.方式一 通过form表单进行文件上传 #=================================== ...