💈 线程间互访助手类 (EN)
Conmajia © 2012, 2018
Published on August 5th, 2012
Updated on February 2nd, 2019
Introduction
While working on background threads, you may encounter updating values of frontend GUI controls. Then you probably will face one particular problem: invalid operation between threads. As shown in figure 1, this issue throws an InvalidOperationException
. It occurs every time when accesses properties/methods from other threads but the one which owns them.
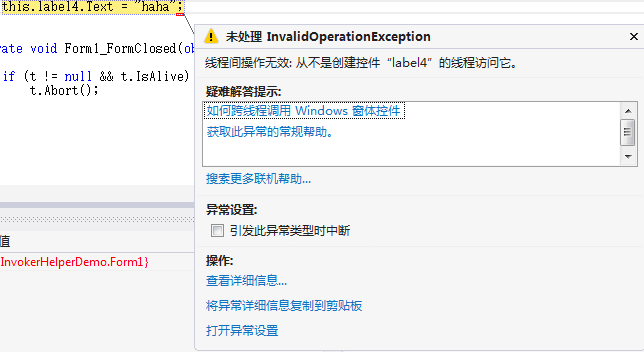
In .NET Framework, version 2.0 as I refer, every Control
class contains an InvokeRequired
property and an Invoke
method to accomplish cross-thread operations. Some typical call code is listed below.
public void DoWork() {
if (control.InvokeRequired) {
control.Invoke(DoWork);
}
else {
// do work
}
}
My Approach
I wrote a helper class InvokeHelper
which granted me the power to access assets between different threads. The class has 3 methods:
Invoke()
- to call methods of a control.
InvokeHelper.Invoke(<control>, "<method>"[, <param1>[,<param2>,...]]);
Get()
- to get properties of a control.
InvokeHelper.Get(<control>, "<property>");
Set()
- to set properties of a control.
InvokeHelper.Set(<control>, "<property>", <value>);
Demonstration
In the demo, I used a forever looping background thread (t
) to show how the InvokeHelper
helps threads to interact. Thread t
updates the frontend thread (the GUI) every 500 milliseconds.
Thread t;
private void button1_Click(object sender, EventArgs e) {
if(t == null) {
t = new Thread(multithread);
t.Start();
label4.Text = string.Format("Thread state:\n{0}", t.ThreadState.ToString());
}
}
public void DoWork(string msg) {
this.label3.Text = string.Format("Invoke method: {0}", msg);
}
int count = 0;
void multithread() {
while(true) {
InvokeHelper.Set(this.label1, "Text", string.Format("Set value: {0}", count));
InvokeHelper.Set(this.label1, "Tag", count);
string value = InvokeHelper.Get(this.label1, "Tag")
.ToString();
InvokeHelper.Set(this.label2, "Text", string.Format("Get value: {0}", value));
InvokeHelper.Invoke(this, "DoWork", value);
Thread.Sleep(500);
count++;
}
}
Results shown in animated figure 2. Obviously, the frontend is not blocked despite t
never stops.
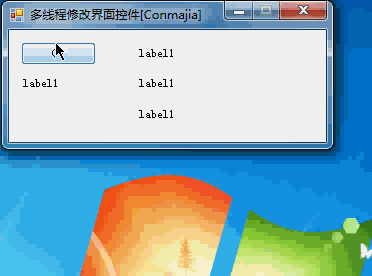
InvokeHelper
Demonstration (animation)Other Approaches
There is a built-in option in the VisualStudio IDE that disables the cross-thread access check: CheckForIllegalCrossThreadCalls
. I found it slightly slower than my helper class. Figure 3 shows the test result.
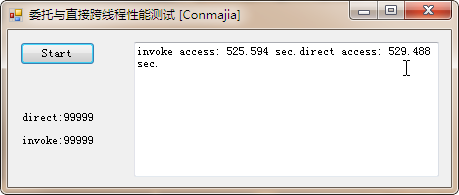
CheckForIllegalCrossThreadCalls
The whole procedure of the test is recorded in figure 4. (The video lasts for 8'51")
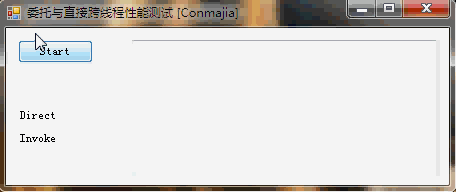
CheckForIllegalCrossThreadCalls
(8'51")Appendix
Source code of the InvokeHelper
is listed below. Zipped .NET project files: Download
public class InvokeHelper {
private delegate object MethodInvoker(Control control, string methodName, params object[] args);
private delegate object PropertyGetInvoker(Control control, object noncontrol, string propertyName);
private delegate void PropertySetInvoker(Control control, object noncontrol, string propertyName, object value);
private static PropertyInfo GetPropertyInfo(Control control, object noncontrol, string propertyName) {
if (control != null && !string.IsNullOrEmpty(propertyName)) {
PropertyInfo pi = null;
Type t = null;
if (noncontrol != null) t = noncontrol.GetType();
else t = control.GetType();
pi = t.GetProperty(propertyName);
if (pi == null) throw new InvalidOperationException(string.Format("Can't find property {0} in {1}.", propertyName, t.ToString()));
return pi;
} else throw new ArgumentNullException("Invalid argument.");
}
public static object Invoke(Control control, string methodName, params object[] args) {
if (control != null && !string.IsNullOrEmpty(methodName))
if (control.InvokeRequired) return control.Invoke(new MethodInvoker(Invoke), control, methodName, args);
else {
MethodInfo mi = null;
if (args != null && args.Length > 0) {
Type[] types = new Type[args.Length];
for (int i = 0; i
References
- Sergiu Josan, Making Controls Thread-safely, May 2009
- vicoB, Extension of safeInvoke, July 2010
The End. \(\Box\)
💈 线程间互访助手类 (EN)的更多相关文章
- Java:多线程,使用同步锁(Lock)时利用Condition类实现线程间通信
如果程序不使用synchronized关键字来保证同步,而是直接使用Lock对象来保证同步,则系统中不存在隐式的同步监视器,也就不能用wait().notify().notifyAll()方法进行线程 ...
- 并发工具类(四)线程间的交换数据 Exchanger
前言 JDK中为了处理线程之间的同步问题,除了提供锁机制之外,还提供了几个非常有用的并发工具类:CountDownLatch.CyclicBarrier.Semphore.Exchanger.Ph ...
- Java并发工具类之线程间数据交换工具Exchanger
Exchanger是一个用于线程间协做的工具类,主要用于线程间的数据交换.它提供了一个同步点,在这个同步点,两个线程可以彼此交换数据.两个线程通过exchange方法交换数据,如果一个线程执行exch ...
- Java并发工具类(四):线程间交换数据的Exchanger
简介 Exchanger(交换者)是一个用于线程间协作的工具类.Exchanger用于进行线程间的数据交换.它提供一个同步点,在这个同步点两个线程可以交换彼此的数据.这两个线程通过exchange方法 ...
- Object类中wait带参方法和notifyAll方法和线程间通信
notifyAll方法: 进入到Timed_Waiting(计时等待)状态有两种方式: 1.sleep(long m)方法,在毫秒值结束之后,线程睡醒,进入到Runnable或BLocked状态 2. ...
- Object类中wait代餐方法和notifyAll方法和线程间通信
Object类中wait代餐方法和notifyAll方法 package com.yang.Test.ThreadStudy; import lombok.SneakyThrows; /** * 进入 ...
- Disruptor——一种可替代有界队列完成并发线程间数据交换的高性能解决方案
本文翻译自LMAX关于Disruptor的论文,同时加上一些自己的理解和标注.Disruptor是一个高效的线程间交换数据的基础组件,它使用栅栏(barrier)+序号(Sequencing)机制协调 ...
- 0038 Java学习笔记-多线程-传统线程间通信、Condition、阻塞队列、《疯狂Java讲义 第三版》进程间通信示例代码存在的一个问题
调用同步锁的wait().notify().notifyAll()进行线程通信 看这个经典的存取款问题,要求两个线程存款,两个线程取款,账户里有余额的时候只能取款,没余额的时候只能存款,存取款金额相同 ...
- 线程间通信 GET POST
线程间通信有三种方法:NSThread GCD NSOperation 进程:操作系统里面每一个app就是一个进程. 一个进程里面可以包含多个线程,并且我们每一个app里面有且仅有一 ...
随机推荐
- Android 画文字图
画图 private Bitmap getbitmap(String content) { Bitmap bitmap = Bitmap.createBitmap(400, 400, Bitmap.C ...
- Windows上安装配置SSH教程(1)——知识点汇总
1.是什么SSH? 维基百科:https://zh.wikipedia.org/wiki/Secure_Shell 其他博客:http://www.ruanyifeng.com/blog/2011/1 ...
- 【转】APP功能测试要领
也许大家从事APP功能测试已经有一段时间了,心中一定有一个疑问,怎么样才能提高测试的覆盖面呢,我今天把APP功能测试内容分为APP本身的功能,APP关联的事务.APP外部环境.APP其他四大块来给大家 ...
- python——在文件存放路径下自动创建文件夹!
1.a.py文件存放的路径下为(D:\Auto\eclipse\workspace\Testhtml\Test) 2.通过os.getcwd()获取的路径为:D:\Auto\eclipse\works ...
- 10位时间戳使用moment转化为日期
前情提要: 需要把后台传过来的10位时间戳转化格式为:‘YYYY-MM-DD HH:mm:ss’的日期展示在页面上.本来是自己写了个函数,但是奈何leader说我们项目用了moment了,你为什么不用 ...
- Vue 进阶之路(七)
之前的文章我们对 vue 的列表输出做了介绍,本章我们来看一下 vue 的组件 component. <!DOCTYPE html> <html lang="en" ...
- TensorFlow从1到2(四)时尚单品识别和保存、恢复训练数据
Fashion Mnist --- 一个图片识别的延伸案例 在TensorFlow官方新的教程中,第一个例子使用了由MNIST延伸而来的新程序. 这个程序使用一组时尚单品的图片对模型进行训练,比如T恤 ...
- Asp.Net Core中HttpClient的使用方式
在.Net Core应用开发中,调用第三方接口也是常有的事情,HttpClient使用人数.使用频率算是最高的一种了,在.Net Core中,HttpClient的使用方式随着版本的升级也发生了一些变 ...
- 给女朋友讲解什么是Optional【JDK 8特性】
前言 只有光头才能变强 前两天带女朋友去图书馆了,随手就给她来了一本<与孩子一起学编程>的书,于是今天就给女朋友讲解一下什么是Optional类. 至于她能不能看懂,那肯定是看不懂的.(学 ...
- canvas百分比加载动画
window.onload = function(){ var canvas = document.getElementById('canvas'), //获取canvas元素 context = c ...