[ACM] HDU 5078 Osu!
Osu!
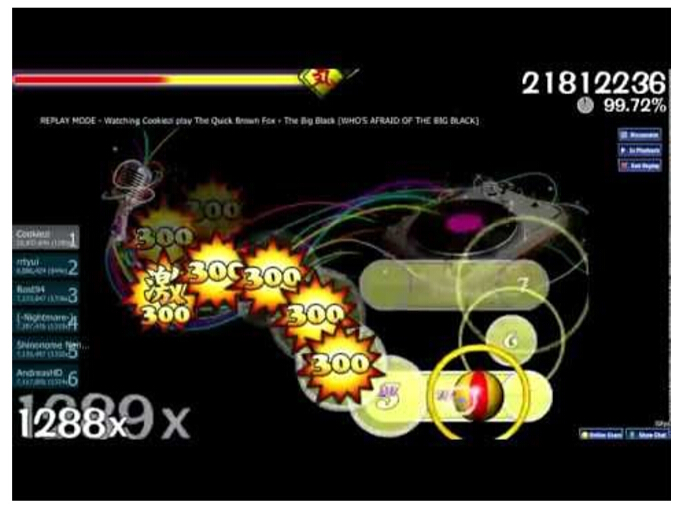
Now, you want to write an algorithm to estimate how diffecult a game is.
To simplify the things, in a game consisting of N points, point i will occur at time ti at place (xi, yi), and you should click it exactly at ti at (xi, yi). That means you should move your cursor
from point i to point i+1. This movement is called a jump, and the difficulty of a jump is just the distance between point i and point i+1 divided by the time between ti and ti+1. And the difficulty of a game is simply the difficulty
of the most difficult jump in the game.
Now, given a description of a game, please calculate its difficulty.
For each test case, the first line contains an integer N (2 ≤ N ≤ 1000) denoting the number of the points in the game. Then N lines follow, the i-th line consisting of 3 space-separated integers, ti(0 ≤ ti < ti+1 ≤ 106),
xi, and yi (0 ≤ xi, yi ≤ 106) as mentioned above.
Your answer will be considered correct if and only if its absolute or relative error is less than 1e-9.
2
5
2 1 9
3 7 2
5 9 0
6 6 3
7 6 0
10
11 35 67
23 2 29
29 58 22
30 67 69
36 56 93
62 42 11
67 73 29
68 19 21
72 37 84
82 24 98
9.2195444573
54.5893762558HintIn memory of the best osu! player ever Cookiezi.
解题思路:
水题,看懂题意,写代码就没问题。
代码:
#include <iostream>
#include <stdio.h>
#include <algorithm>
#include <string.h>
#include <cmath>
#include <iomanip>
#include <vector>
#include <map>
#include <stack>
#include <queue>
using namespace std;
int n; struct Point
{
int x,y,t;
}point[1002]; double dis(Point a,Point b)
{
return sqrt((double)(a.x-b.x)*(a.x-b.x)+(double)(a.y-b.y)*(a.y-b.y));
} int main()
{
int t;cin>>t;
while(t--)
{
cin>>n;
cin>>point[1].t>>point[1].x>>point[1].y;
double ans=-1;
for(int i=2;i<=n;i++)
{
cin>>point[i].t>>point[i].x>>point[i].y;
double temp=dis(point[i],point[i-1])/(point[i].t-point[i-1].t);
if(ans<temp)
ans=temp;
}
cout<<setiosflags(ios::fixed)<<setprecision(9)<<ans<<endl;
}
return 0;
}
[ACM] HDU 5078 Osu!的更多相关文章
- hdu 5078 Osu! (2014 acm 亚洲区域赛鞍山 I)
题目链接:http://acm.hdu.edu.cn/showproblem.php? pid=5078 Osu! Time Limit: 2000/1000 MS (Java/Others) ...
- 2014 Asia AnShan Regional Contest --- HDU 5078 Osu!
Osu! Problem's Link: http://acm.hdu.edu.cn/showproblem.php?pid=5078 Mean: 略. analyse: 签到题,直接扫一遍就得答 ...
- hdu 5078 Osu!(鞍山现场赛)
Osu! Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 262144/262144 K (Java/Others) Total Sub ...
- hdu 5078 2014鞍山现场赛 水题
http://acm.hdu.edu.cn/showproblem.php?pid=5078 现场最水的一道题 连排序都不用,由于说了ti<ti+1 //#pragma comment(link ...
- HDU 4911 http://acm.hdu.edu.cn/showproblem.php?pid=4911(线段树求逆序对)
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=4911 解题报告: 给出一个长度为n的序列,然后给出一个k,要你求最多做k次相邻的数字交换后,逆序数最少 ...
- KMP(http://acm.hdu.edu.cn/showproblem.php?pid=1711)
http://acm.hdu.edu.cn/showproblem.php?pid=1711 #include<stdio.h> #include<math.h> #inclu ...
- HDU-4632 http://acm.hdu.edu.cn/showproblem.php?pid=4632
http://acm.hdu.edu.cn/showproblem.php?pid=4632 题意: 一个字符串,有多少个subsequence是回文串. 别人的题解: 用dp[i][j]表示这一段里 ...
- ACM HDU 1559 最大子矩阵
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=1559 这道题 挺好的,当时想出解法的时候已经比较迟了.还是平时看得少. 把行与列都进行压缩.ans[i ...
- ACM HDU Bone Collector 01背包
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=2602 这是做的第一道01背包的题目.题目的大意是有n个物品,体积为v的背包.不断的放入物品,当然物品有 ...
随机推荐
- django返回响应对象
Django的视图必须要返回一个HttpResponse对象(或者其子类对象),不能像flask一样直接返回字符串. Django: return HttpResponse("Hello&q ...
- springBoot Ribbon Hystrix Dashboard
1.引入依赖 <!-- 引入关于 hystrix Dashboard的依赖 --> <dependency> <groupId>org.springframewor ...
- AC日记——[SCOI2008] 着色方案 bzoj 1079
1079 思路: dp: 我们如果dp方程为15维,每维记录颜色还有多少种: 不仅tle,mle,它还re: 所以,我们压缩一下dp方程: 方程有6维,第i维记录有多少种颜色还剩下i次: 最后还要记录 ...
- react setState里的作用域
从接触racet开始,我们就认识了setState,它是对全局变量进去更新的一个重要方法, 不仅可以更新数据,还能在更新后执行方法时直接调用刚刚更新的数据 今天碰到的问题就在于它的作用域的先后问题 先 ...
- Codeforces Beta Round #4 (Div. 2 Only) A. Watermelon【暴力/数学/只有偶数才能分解为两个偶数】
time limit per test 1 second memory limit per test 64 megabytes input standard input output standard ...
- 【高精度】高精度数除以低精度数I
问题 G: [高精度]高精度数除以低精度数I 时间限制: 1 Sec 内存限制: 512 MB提交: 173 解决: 71[提交] [状态] [讨论版] [命题人:] 题目描述 修罗王聚集了庞大的 ...
- Union与UnionAll
UNION指令的目的是将两个SQL语句的结果合并起来.从这个角度来看, 我们会产生这样的感觉,UNION跟JOIN似乎有些许类似,因为这两个指令都可以由多个表格中撷取资料. UNION的一个限制是两个 ...
- 【bzoj4950】【 [Wf2017]Mission Improbable】贪心+二分图匹配
(上不了p站我要死了,侵权度娘背锅) Description 那是春日里一个天气晴朗的好日子,你准备去见见你的老朋友Patrick,也是你之前的犯罪同伙.Patrick在编程竞赛 上豪赌输掉了一大笔钱 ...
- [置顶]
kubernetes--Init Container
概念 Init Container就是做初始化工作的容器.可以有一个或多个,如果有多个,这些 Init Container 按照定义的顺序依次执行,只有所有的InitContainer 执行完后,主容 ...
- CURL简单使用
学习地址:https://yq.aliyun.com/articles/33262 curl的简单使用步骤 要使用cURL来发送url请求,具体步骤大体分为以下四步: 1.初始化2.设置请求选项3.执 ...