Android 之http编程
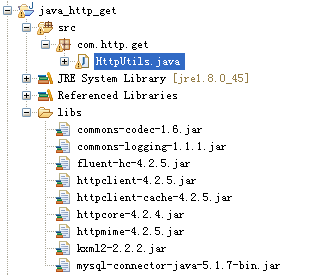
源码:
package com.http.get; import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL; public class HttpUtils { private static String urlPath="http://i6.topit.me/6/5d/45/1131907198420455d6o.jpg";
private static HttpURLConnection httpURLConnection=null;
public HttpUtils() {
// TODO Auto-generated constructor stub
}
public static InputStream getInputStream() throws IOException{
InputStream inputStream=null;
URL url=new URL(urlPath);
if(url!=null){
httpURLConnection=(HttpURLConnection)url.openConnection();
httpURLConnection.setConnectTimeout();
httpURLConnection.setDoInput(true);
httpURLConnection.setRequestMethod("GET");
int code=httpURLConnection.getResponseCode();
if(code==){
inputStream=httpURLConnection.getInputStream();
}
}
return inputStream;
}
public static void getImgToDisk() throws IOException{
InputStream inputStream=getInputStream();
FileOutputStream fileOutputStream=new FileOutputStream("D:\\TEST.png");
byte[] data=new byte[];
int len=;
while((len=inputStream.read(data))!=-){
fileOutputStream.write(data, , len);
}
if(inputStream!=null)
inputStream.close();
if(fileOutputStream!=null)
fileOutputStream.close();
}
public static void main(String[] args) throws IOException {
getImgToDisk();
}
}
案例2:以Java接口POST方式向服务器提交用户名和密码,在服务器端进行简单判断,如果正确返回字符串success,否则返回fail
服务器端简单校验:
*/
public void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/html;charset=utf-8");
request.setCharacterEncoding("utf-8");
response.setCharacterEncoding("utf-8"); PrintWriter out = response.getWriter();
//String jsonString =JsonTools.createJsonString("person", service.getPerson());
String name = request.getParameter("username");
String pwd = request.getParameter("password");
if (name.equals("admin")&&pwd.equals("")) {
out.print("success");
}
else {
out.print("fail");
}
out.flush();
out.close();
}
客户端的提交:
package com.http.post;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.UnsupportedEncodingException;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import java.net.URLEncoder;
import java.util.HashMap;
import java.util.Map; import sun.net.www.http.HttpClient; public class HttpUtils { private static String PATH="http://122.206.79.193:8080/myhttp/servlet/LoginAction";
private static URL URL;
static{
try {
URL=new URL(PATH);
} catch (MalformedURLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
public HttpUtils() {
// TODO Auto-generated constructor stub
} /**
*
* @param params 填写的参数
* @param encode 字节编码 为了避免乱码
* @return 从服务端返回的信息
* @throws Exception
*/
public static String sendPostMessage(Map<String,String>params,String encode) throws Exception{
StringBuffer sb=new StringBuffer();
if(params!=null&&!params.isEmpty()){
for(Map.Entry<String,String> entry:params.entrySet()){
sb.append(entry.getKey()).append("=").append(URLEncoder.encode(entry.getValue(),encode)).append("&");
}
sb.deleteCharAt(sb.length()-);
HttpURLConnection httpURLConnection=(HttpURLConnection)URL.openConnection();
httpURLConnection.setConnectTimeout();
httpURLConnection.setDoInput(true);//从服务器获取数据
httpURLConnection.setDoOutput(true);//向服务器提交数据
//获得上传信息字节大小及长度
byte[] data=sb.toString().getBytes();
//浏览器内置封装了http协议信息,但是手机或者用Java访问时并没有 所以最重要的是需要设置请求体的属性封装http协议
//设置请求体的请求类型是文本类型
httpURLConnection.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");//文本类型
//设置请求体的长度
httpURLConnection.setRequestProperty("Content-Length",String.valueOf(data.length));
//想要向服务器提交数据必须设置输出流,向服务器输出数据
System.out.println(httpURLConnection.toString());
OutputStream out=httpURLConnection.getOutputStream();
out.write(data, , data.length);
out.close();
int code=httpURLConnection.getResponseCode();
if(code==){
return changeInputStream(httpURLConnection.getInputStream(),encode);
} }
return "";
}
private static String changeInputStream(InputStream inputStream,String encode) {
// TODO Auto-generated method stub
String resString = "";
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();//内存流
int len = ;
byte[] data = new byte[];
try {
while ((len = inputStream.read(data)) != -) {
outputStream.write(data, , len);
}
resString = new String(outputStream.toByteArray());
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return resString;
}
public static void main(String[] args) throws Exception {
Map<String,String>params=new HashMap<String, String>();
params.put("username", "admin");
params.put("password", "");
String res=sendPostMessage(params,"utf-8");
System.out.println("-res->>"+res);
}
}
标准Apache接口
package com.http.post; import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.UnsupportedEncodingException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map; import org.apache.http.HttpResponse;
import org.apache.http.NameValuePair;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.message.BasicNameValuePair; public class HttpUtils { public HttpUtils() {
// TODO Auto-generated constructor stub
}
public static String sendHttpClientPost(String path,Map<String,String>map,String encode) throws Exception, IOException{ //将参数map进行封装到list中
List<NameValuePair> list=new ArrayList<NameValuePair>();
if(map!=null&&!map.isEmpty()){
for(Map.Entry<String, String> entry:map.entrySet()){
list.add(new BasicNameValuePair(entry.getKey(), entry.getValue()));
}
}
try {
//将请求的参数封装的list到表单,即请求体中
UrlEncodedFormEntity entity=new UrlEncodedFormEntity(list,encode);
//使用post方式提交数据
HttpPost httpPost=new HttpPost(path);
httpPost.setEntity(entity);
DefaultHttpClient client=new DefaultHttpClient();
HttpResponse response= client.execute(httpPost);
//以上完成提交
//以下完成读取服务端返回的信息
if(response.getStatusLine().getStatusCode()==){
return changeInputStream(response.getEntity().getContent(),encode);
}
} catch (UnsupportedEncodingException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
public static String changeInputStream(InputStream inputStream,String encode) {
// TODO Auto-generated method stub
String resString = "";
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();//内存流
int len = ;
byte[] data = new byte[];
try {
while ((len = inputStream.read(data)) != -) {
outputStream.write(data, , len);
}
resString = new String(outputStream.toByteArray());
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return resString;
}
public static void main(String[] args) throws Exception, Exception {
String path="http://localhost:8080/myhttp/servlet/LoginAction";
Map<String,String>map=new HashMap<String, String>();
map.put("username", "admin");
map.put("password", "");
String msg=HttpUtils.sendHttpClientPost(path, map, "utf-8");
System.out.println("-->>--"+msg);
} }
Android 之http编程的更多相关文章
- Android中JNI编程的那些事儿(1)
转:Android中JNI编程的那些事儿(1)http://mobile.51cto.com/android-267538.htm Android系统不允许一个纯粹使用C/C++的程序出现,它要求必须 ...
- Android 框架式编程 —— 起篇
一般的,在开发的时候,写过的代码在需求变更后,发现需要改动非常多的地方,那么说明之前的代码的架构肯定是存在问题的. 下面我们结合面向对象的六大基本原则谈Android 框架式编程.首先先介绍一下面向对 ...
- Android 的网络编程
android的网络编程分为2种:基于socket的,和基于http协议的. 基于socket的用法 服务器端: 先启动一个服务器端的socket ServerSocket svr = new ...
- Android手机摄像头编程入门
本讲内容:Android手机摄像头编程入门智能手机中的摄像头和普通手机中的摄像头最大的区别在于,智能机上的摄像头可以由程序员写程序控制, 做一些有趣的应用譬如,画中画,做一些有用的应用譬如二维码识别, ...
- 《Android传感器高级编程》
<Android传感器高级编程> 基本信息 原书名:Professional Android Sensor Programming 原出版社: Wrox 作者: (美)米内特(Greg M ...
- (转载)android开发常见编程错误总结
首页 › 安卓开发 › android开发 android开发常见编程错误总结 泡在网上的日子 / 文 发表于2013-09-07 13:07 第771次阅读 android,异常 0 编辑推荐:稀 ...
- Android Studio NDK编程初探
继上一篇学习了如何使用NDK编译FFMPEG后,接下来就是要学习如何在Android Studio中使用了. 经过参考和一系列的摸索,记录下具体步骤. 创建C++ Support的Android St ...
- (转)android 蓝牙通信编程
转自:http://blog.csdn.net/pwei007/article/details/6015907 Android平台支持蓝牙网络协议栈,实现蓝牙设备之间数据的无线传输. 本文档描述了怎样 ...
- Android BLE 蓝牙编程(三)
上节我们已经可以连接上蓝牙设备了. 本节我们就要获取手环的电池电量和计步啦. 在介绍这个之前我们需要先了解下什么是 服务 什么是 UUID 我们记得上节中我们item监听事件的回调的返回值是Bluet ...
- Android first --- 网络编程
网络编程 ###图片下载查看 1.发送http请求 URL url = new URL(address); //获取连接对象,并没有建立连接 HttpURLConnection conn = (Htt ...
随机推荐
- scope引起的问题
背景 执行mvn clean test命令提示部分包不存在,但通过eclipse的clean操作后可以执行mvn test命令 解决方法 mvn clean操作为清空编译的class文件,test的话 ...
- Win7怎么把运行添加到Win快捷菜单的右侧、、、
win7怎么把运行添加到Win快捷菜单的右侧... ------------------------------ 右键桌面任务栏--选择属性 ----------------------------- ...
- 大数据平台搭建-hadoop/hbase集群的搭建
版本要求 java 版本:1.8.*(1.8.0_60) 下载地址:http://www.oracle.com/technetwork/java/javase/downloads/jdk8-downl ...
- Tornado-数据库(torndb包)
1.torndb数据库简介 在Tornado3.0版本以前提供tornado.database模块用来操作MySQL数据库,而从3.0版本开始,此模块就被独立出来,作为torndb包单独提供. ...
- javascript学习笔记-3
1.对于javascript中的this关键字,表示的是当前代码所处的对象. var a={ get:function(){ this.val=12 } } console.log(a.val); a ...
- Spring 设置readonly 事务只读模式
详见:http://blog.yemou.net/article/query/info/tytfjhfascvhzxcyt394 在学习spring事务的时候,发现设置readOnly后不启作用. 查 ...
- 常用按钮css
#openwx_btn { border: 0px; background-color: rgb(145, ...
- C# IComparable 和 IComparer 区别
理解很重要: 开始对这两个接口的区别一直是很模糊,看到很多书后,终于知道了区别,形成了个人的理解: 关于 IComparable 比喻一个类person实现了 IComparable,那么它就要重写C ...
- 新CCIE笔记之'口口相传'路由协议
//由于思科所有命令行中没有尖括号"<>"这样的关键字,所以本文中出现命令行中的尖括号中的内容均为注释提示信息,代表此处应该填入那一类数据. 请容许我将RIP和EIGR ...
- 微信小程序icon,text,progress标签的测试
一:testIconAndTextAndProgress.wxml的代码如下.testIconAndTextAndProgress.js自动生成示例代码 //testIconAndTextAndPro ...